static std::string INPUTPATH;
时间: 2024-08-13 19:07:15 浏览: 139
`static std::string INPUTPATH;` 是 C++ 中的一个声明,用于定义一个静态字符串变量。这里有几个关键点:
1. **Static**: "static" 关键字意味着这个变量的生命周期与整个程序同寿命,即只会在程序启动时初始化一次,不会因为函数调用结束而销毁。这样可以在整个程序中共享同一个输入路径。
2. **std::string**: "std::string" 是 C++ 标准库中的字符串类型,用于存储文本数据。
3. **INPUTPATH**: 这是一个变量名,可能被用作读取或处理某个输入文件路径的占位符。
具体用途可能是在读取配置文件、命令行参数或用户输入时,存储默认或用户指定的输入文件路径。相关问题:
相关问题
如何实现一个test(std::string path_int, std::string path_out)jni接口和test_callback(std::string path_out)jni接口
要实现一个test(std::string path_int, std::string path_out) JNI接口和test_callback(std::string path_out) JNI接口,需要按照以下步骤进行操作:
1.创建一个C++头文件,并在其中声明test(std::string path_int, std::string path_out)和test_callback(std::string path_out) JNI接口函数。
2.在C++源文件中实现这些JNI接口函数,并包含jni.h头文件以便使用JNI API。
3.编写Java类,并使用Java Native Interface (JNI)来访问C++代码。
4.在Java类中声明native方法,并使用JNI方法注册这些native方法。
5.在Java代码中实现这些native方法,以便在C++代码中调用。
以下是一个简单的示例:
C++头文件:
```
#ifndef _MY_JNI_INTERFACE_H_
#define _MY_JNI_INTERFACE_H_
#include <jni.h>
#include <string>
#ifdef __cplusplus
extern "C" {
#endif
JNIEXPORT void JNICALL Java_com_example_MyClass_test(JNIEnv* env, jobject obj, jstring path_int, jstring path_out);
JNIEXPORT void JNICALL Java_com_example_MyClass_test_1callback(JNIEnv* env, jobject obj, jstring path_out);
#ifdef __cplusplus
}
#endif
#endif // _MY_JNI_INTERFACE_H_
```
C++源文件:
```
#include "my_jni_interface.h"
JNIEXPORT void JNICALL Java_com_example_MyClass_test(JNIEnv* env, jobject obj, jstring path_int, jstring path_out) {
const char* c_path_int = env->GetStringUTFChars(path_int, NULL);
const char* c_path_out = env->GetStringUTFChars(path_out, NULL);
// 实现test函数的代码
env->ReleaseStringUTFChars(path_int, c_path_int);
env->ReleaseStringUTFChars(path_out, c_path_out);
}
JNIEXPORT void JNICALL Java_com_example_MyClass_test_1callback(JNIEnv* env, jobject obj, jstring path_out) {
const char* c_path_out = env->GetStringUTFChars(path_out, NULL);
// 实现test_callback函数的代码
env->ReleaseStringUTFChars(path_out, c_path_out);
}
```
Java类:
```
package com.example;
public class MyClass {
static {
System.loadLibrary("my_jni_interface");
}
public native void test(String path_int, String path_out);
public native void test_callback(String path_out);
}
```
JNI方法注册:
```
#include <jni.h>
#include "my_jni_interface.h"
JNIEXPORT jint JNICALL JNI_OnLoad(JavaVM* vm, void* reserved) {
JNIEnv* env = NULL;
jint result = -1;
if (vm->GetEnv((void**)&env, JNI_VERSION_1_6) != JNI_OK) {
return JNI_ERR;
}
jclass clazz = env->FindClass("com/example/MyClass");
if (clazz == NULL) {
return JNI_ERR;
}
JNINativeMethod methods[] = {
{"test", "(Ljava/lang/String;Ljava/lang/String;)V", (void*)Java_com_example_MyClass_test},
{"test_callback", "(Ljava/lang/String;)V", (void*)Java_com_example_MyClass_test_1callback}
};
if (env->RegisterNatives(clazz, methods, sizeof(methods) / sizeof(methods[0])) < 0) {
return JNI_ERR;
}
return JNI_VERSION_1_6;
}
```
Java代码:
```
package com.example;
public class Main {
public static void main(String[] args) {
MyClass myClass = new MyClass();
String path_int = "/path/to/input/file";
String path_out = "/path/to/output/file";
myClass.test(path_int, path_out);
myClass.test_callback(path_out);
}
}
```
这样,当Java代码调用test和test_callback方法时,将会执行C++实现的test和test_callback JNI接口函数。
阅读全文
相关推荐
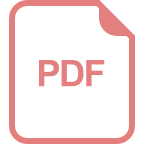
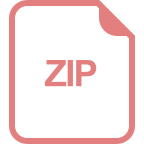
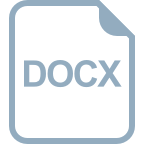
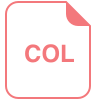
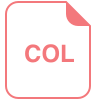
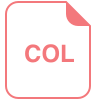
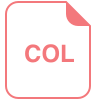
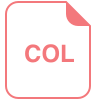
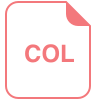
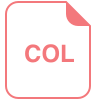
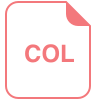
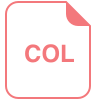
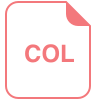
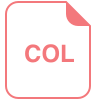
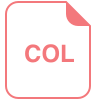
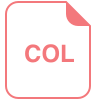
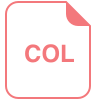
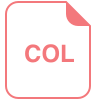