action = self.sess.run(self.actor_net.output, feed_dict={self.state_ph: state.reshape(1, 2)})[0] # Add batch dimension to single state input, and remove batch dimension from single action output AttributeError: 'list' object has no attribute 'reshape'
时间: 2024-03-17 14:43:32 浏览: 23
这个错误通常是因为代码中使用了一个列表对象,而列表对象没有 reshape() 方法,导致出现了 AttributeError 错误。具体来说,错误提示 "'list' object has no attribute 'reshape'" 表示列表对象没有 reshape() 方法,因此无法执行这个操作。
在这段代码中,state 变量可能是一个列表对象,而不是一个 numpy 数组。解决这个问题的方法是将 state 变量转换为 numpy 数组,然后再调用 reshape() 方法。可以使用以下代码来解决这个问题:
```
state = np.array(state)
action = self.sess.run(self.actor_net.output, feed_dict={self.state_ph: state.reshape(1, 2)})[0]
```
这里使用 numpy 的 array() 函数将 state 转换为 numpy 数组,然后再调用 reshape() 方法将其形状改为 (1, 2)。这样就可以避免 "'list' object has no attribute 'reshape'" 的 AttributeError 错误。
除了上述方法,还可以使用其他将列表对象转换为 numpy 数组的方法,例如 np.asarray() 函数。需要根据具体情况选择合适的方法。
相关问题
action = self.sess.run(self.actor_net.output, state)[0]
这段代码的作用是使用actor网络对给定的状态state进行预测,输出对应的动作action。具体来说,self.actor_net是一个神经网络模型,它的输入是状态state,输出是动作action。self.actor_net.output表示神经网络的输出层,它对应的张量的形状通常是(1, action_dim),其中action_dim是动作的维度。self.sess.run(self.actor_net.output, state)表示将状态state输入到神经网络中进行计算,得到输出层的结果,即对应的动作action。由于action是一个长度为action_dim的一维数组,因此我们通过action[0]来获取其中的一个元素,即最终输出的动作。
action = self.sess.run(self.actor_net.output, {self.state_ph:np.expand_dims(1, 2)})
这段代码中的 np.expand_dims() 函数的参数应该是 2,而不是 0。这个函数的作用是将 1 转换为一个形状为 (1,1) 的 numpy 数组,然后将其传递给 self.state_ph 占位符,该占位符期望接收一个形状为 (None,state_dim) 的数据,其中 None 表示可以接受任意数量的状态数据。因此,这里可能会引发一个形状不匹配的错误。另外,使用 [0] 将结果转换为形状为 (action_dim,) 的 numpy 数组的方法是不正确的,因为这个操作会将结果的第一个元素提取出来,而不是将整个结果的维度降低。如果想要将结果的维度降低,可以使用 np.squeeze() 函数,例如:
```
action = self.sess.run(self.actor_net.output, {self.state_ph: np.expand_dims(1, 2)})
action = np.squeeze(action, axis=0)
```
这个代码段将 self.actor_net 模型的输出作为 action,并将形状为 (1,1,action_dim) 的 numpy 数组使用 np.squeeze() 函数转换为形状为 (action_dim,) 的 numpy 数组。这样,得到的 action 就是当前状态下模型预测的动作。
相关推荐
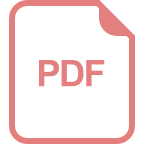
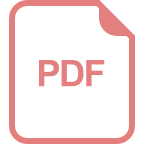
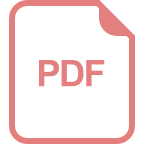













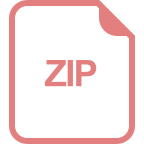