springboot3.0如何集成springSecurity
时间: 2023-09-06 15:05:27 浏览: 148
### 回答1:
SpringBoot 3.0可以使用Spring Security来实现安全认证和授权控制,可以通过在Spring Boot项目的Maven依赖中添加spring-boot-starter-security依赖来实现。
### 回答2:
要集成Spring Security到Spring Boot 3.0中,可以按照以下步骤操作:
1. 在pom.xml文件中添加Spring Security的依赖项:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
2. 创建一个配置类,例如SecurityConfig,该类需要继承自WebSecurityConfigurerAdapter,并使用@EnableWebSecurity注解启用Spring Security配置。
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/public/**").permitAll() // 设置公开访问的URL
.anyRequest().authenticated() // 其他URL需要认证
.and()
.formLogin() // 使用表单登录
.and()
.logout().permitAll(); // 允许注销
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication() // 使用内存中的用户进行身份认证
.withUser("admin").password("{noop}password").roles("ADMIN")
.and()
.withUser("user").password("{noop}password").roles("USER");
}
}
```
3. 在configure(HttpSecurity http)方法中配置URL的访问权限,设置公开访问的URL和需要认证的URL。这里演示了两个简单的示例,所有以/public/开头的URL都允许公开访问,其他URL需要认证。
4. 在configure(AuthenticationManagerBuilder auth)方法中配置用户的身份认证信息。这里使用了内存中的用户,用户名为admin和user,密码为password,分别具有ADMIN和USER角色。
5. 可以根据自己的需求进行进一步配置,例如添加自定义的登录页面、自定义登录成功/失败的处理逻辑等。
通过以上步骤,就可以将Spring Security集成到Spring Boot 3.0中。需要注意的是,这只是一个简单的示例,实际应用中可能需要根据实际情况进行调整和配置。
### 回答3:
要将Spring Boot 3.0集成Spring Security,需要执行以下步骤:
1. 首先,在项目的pom.xml文件中添加Spring Security的依赖项。可以在Spring Boot官方文档中找到最新版本的依赖项坐标。
2. 创建一个继承自WebSecurityConfigurerAdapter的配置类,并使用@EnableWebSecurity注解标记该类。这个配置类将用于定义安全规则。
3. 在配置类中,覆盖configure(HttpSecurity http)方法来配置安全策略。这个方法允许你定义哪些URL路径需要被保护,哪些路径可以被公开访问,以及如何进行登录认证等。
4. 如果需要自定义登录页面,可以创建一个继承自WebMvcConfigurer的配置类,并覆盖addViewControllers方法来注册自定义登录页面的URL路径。
5. 如果需要自定义认证逻辑,可以创建一个实现UserDetailsService接口的自定义用户服务类,并将其注册为Spring组件。在configure方法中使用userDetailsService()方法来指定这个自定义用户服务类。
6. 可以使用@EnableGlobalMethodSecurity注解来启用全局方法级别的安全性,并设置相关的注解。
7. 最后,可以使用SecurityContextHolder来实现在应用程序中获得当前用户的信息。
通过以上步骤,就可以将Spring Boot 3.0和Spring Security集成起来。这样就可以保护应用程序的URL路径,实现用户认证和授权的功能。
阅读全文
相关推荐
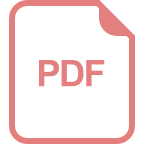
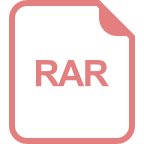


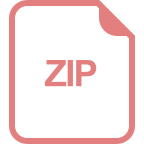

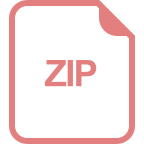


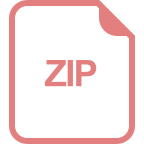
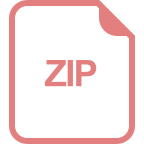
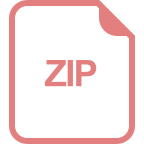
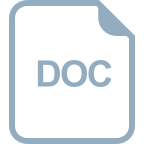
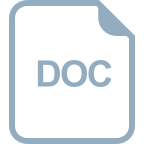



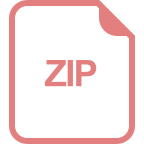