使用c语言编写一段代码,该代码效果是:使串口发送十六位数据,500ms发送0x31,再过500ms发送0x32
时间: 2024-02-17 10:04:03 浏览: 59
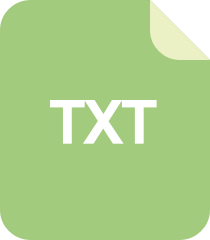
C语言串口发送数据VS2013
下面是一个使用C语言编写的简单串口发送数据的示例代码,可以实现您的需求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd;
struct termios options;
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY); // 初始化串口,根据实际情况修改串口号
if (fd == -1) {
perror("open");
exit(1);
}
fcntl(fd, F_SETFL, 0);
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置波特率
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB; // 设置数据位和停止位
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
tcsetattr(fd, TCSANOW, &options);
while (1) {
write(fd, "\x31", 2); // 发送0x31
usleep(500000); // 等待500毫秒
write(fd, "\x32", 2); // 发送0x32
usleep(500000); // 等待500毫秒
}
close(fd);
return 0;
}
```
以上代码中,我们使用`open`函数打开了串口,并且使用`tcgetattr`和`tcsetattr`函数配置了串口的参数,包括波特率、数据位、停止位等。在一个无限循环中,我们使用`write`函数向串口发送数据,等待500毫秒,然后再发送另一组数据。
请注意,上面的代码仅仅是一个简单的示例,您可能需要根据实际情况进行修改和优化。例如,您可能需要增加一些错误处理代码,以避免程序崩溃或者陷入死循环。
阅读全文
相关推荐
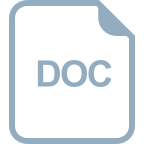
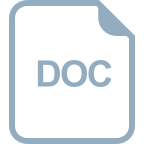
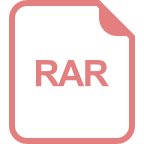
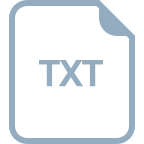
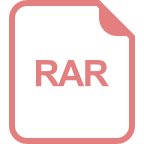
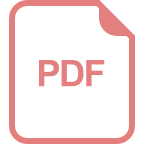
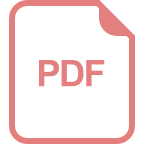
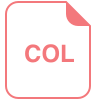
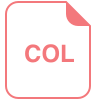
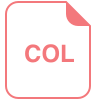
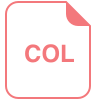
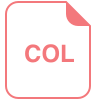
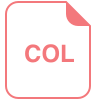
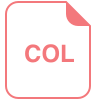
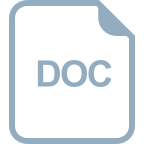
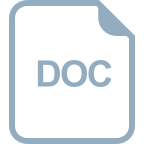
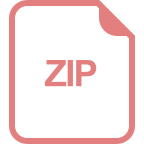