c++中创建一个类使用std::sort对十一个有重复数据的整型变量进行排序,并获取排序后每个变量对应的变量名
时间: 2024-11-27 16:12:28 浏览: 7
在C++中,首先你需要创建一个包含整数及其名称的对象类,例如`ClassWithIntegers`。然后你可以创建一个结构体或者辅助类来存储变量名和值,比如`VariableInfo`。接着,使用`std::vector`来存储这些对象,因为`std::sort`更适合处理动态大小的数组。
```cpp
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
// 定义一个结构体来存储变量名和整数值
struct VariableInfo {
std::string name;
int value;
};
class ClassWithIntegers {
public:
// 添加整数并设置其名字
void addInteger(const std::string& name, int value) {
variable_info.push_back({name, value});
}
// 对变量信息按值排序
void sortIntegers() {
std::sort(variable_info.begin(), variable_info.end(),
[](const VariableInfo& a, const VariableInfo& b) { return a.value < b.value; });
}
// 打印排序后的变量和对应值
void printSortedVariables() {
for (const auto& info : variable_info) {
std::cout << "Variable: " << info.name << ", Value: " << info.value << std::endl;
}
}
private:
std::vector<VariableInfo> variable_info;
};
int main() {
ClassWithIntegers obj;
// 添加一些有重复数据的整数,例如 [5, 2, 7, 2, 9, 5]
obj.addInteger("a", 5);
obj.addInteger("b", 2);
obj.addInteger("c", 7);
obj.addInteger("d", 2);
obj.addInteger("e", 9);
obj.addInteger("f", 5);
// 排序并打印结果
obj.sortIntegers();
obj.printSortedVariables();
return 0;
}
```
在这个例子中,`std::sort`会按照`VariableInfo`中的`value`字段进行升序排列。注意,这里我们假设你已经有了有效的变量名字符串。如果你想从其他地方获取这些名字,你需要提供相应的逻辑。
阅读全文
相关推荐
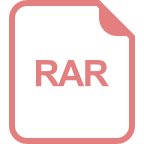
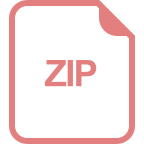
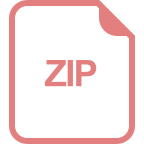




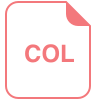
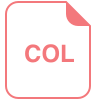
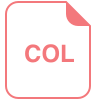
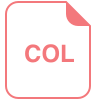
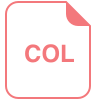
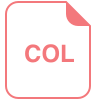
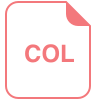
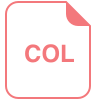
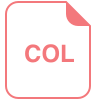
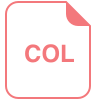
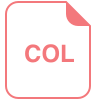
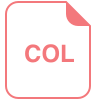