使用javamail查看有附件的邮件
时间: 2024-03-18 20:41:09 浏览: 21
以下是使用 JavaMail API 查看有附件的邮件的示例代码:
```java
import java.io.IOException;
import java.util.Properties;
import javax.mail.BodyPart;
import javax.mail.Folder;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Multipart;
import javax.mail.Session;
import javax.mail.Store;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMultipart;
public class MailAttachmentViewer {
public static void main(String[] args) throws Exception {
Properties properties = new Properties();
properties.put("mail.store.protocol", "imaps");
properties.put("mail.imaps.host", "imap.gmail.com");
properties.put("mail.imaps.port", "993");
properties.put("mail.imaps.starttls.enable", "true");
Session emailSession = Session.getDefaultInstance(properties);
Store store = emailSession.getStore("imaps");
store.connect("your-email@gmail.com", "your-password");
Folder emailFolder = store.getFolder("INBOX");
emailFolder.open(Folder.READ_ONLY);
Message[] messages = emailFolder.getMessages();
for (int i = 0; i < messages.length; i++) {
Message message = messages[i];
System.out.println("Subject: " + message.getSubject());
System.out.println("From: " + message.getFrom()[0]);
Object content = message.getContent();
if (content instanceof Multipart) {
Multipart multipart = (Multipart) content;
for (int j = 0; j < multipart.getCount(); j++) {
BodyPart bodyPart = multipart.getBodyPart(j);
if (bodyPart instanceof MimeBodyPart) {
MimeBodyPart mimeBodyPart = (MimeBodyPart) bodyPart;
if (mimeBodyPart.getDisposition() != null && mimeBodyPart.getDisposition().equalsIgnoreCase("attachment")) {
System.out.println("Attachment found: " + mimeBodyPart.getFileName());
// if you want to download the attachment, use the following code:
// mimeBodyPart.saveFile("/path/to/save/attachment/" + mimeBodyPart.getFileName());
} else {
System.out.println("Text: " + mimeBodyPart.getContent().toString());
}
}
}
} else {
System.out.println("Text: " + content.toString());
}
}
emailFolder.close(false);
store.close();
}
}
```
请注意,如果您想下载附件,您需要取消注释示例代码中的一行,将附件保存到您的本地计算机上。
相关推荐
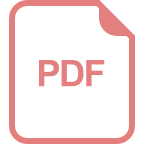














