QAbstractItemModel模拟文件管理器
时间: 2024-01-15 11:05:35 浏览: 29
QAbstractItemModel是Qt框架中的一个抽象类,它提供了一种通用的方式来管理数据,并且可以在可视化控件(如QTreeView、QListView等)中显示这些数据。因此,我们可以使用QAbstractItemModel来模拟文件管理器。
首先,我们需要继承QAbstractItemModel类,实现它的虚函数。在这个模型中,每个项目(item)代表一个文件或目录,我们需要为每个项目存储其名称、路径、类型等信息。
下面是一个简单的示例:
```cpp
class FileSystemModel : public QAbstractItemModel
{
public:
enum class Column {
Name = 0,
Type,
Size,
Path
};
struct FileInfo {
QString name;
QString path;
bool isDir;
qint64 size;
};
explicit FileSystemModel(QObject *parent = nullptr);
~FileSystemModel() override;
QModelIndex index(int row, int column, const QModelIndex &parent = QModelIndex()) const override;
QModelIndex parent(const QModelIndex &child) const override;
int rowCount(const QModelIndex &parent = QModelIndex()) const override;
int columnCount(const QModelIndex &parent = QModelIndex()) const override;
QVariant data(const QModelIndex &index, int role = Qt::DisplayRole) const override;
QVariant headerData(int section, Qt::Orientation orientation, int role = Qt::DisplayRole) const override;
private:
void populateModel(const QString &path, const QModelIndex &parent);
QList<FileInfo> m_fileInfos;
};
```
在这个模型中,我们定义了一个枚举类型Column,表示模型中的列。我们还定义了一个FileInfo结构体,用于存储每个项目的信息。
接下来,我们需要实现QAbstractItemModel类的虚函数。其中,index()和parent()函数用于获取项目的索引和父级项目的索引;rowCount()和columnCount()函数用于获取行数和列数;data()函数用于获取项目的数据;headerData()函数用于获取表头数据。
populateModel()函数用于初始化模型。我们可以在这个函数中遍历指定路径下的所有文件和目录,并将它们添加到模型中。在这个过程中,我们需要记录每个项目的信息,以便在data()函数中返回正确的数据。
下面是一个简化的populateModel()函数:
```cpp
void FileSystemModel::populateModel(const QString &path, const QModelIndex &parent)
{
QDir dir(path);
QFileInfoList entries = dir.entryInfoList(QDir::AllEntries | QDir::NoDotAndDotDot);
for (const QFileInfo &entry : entries) {
FileInfo info;
info.name = entry.fileName();
info.path = entry.absoluteFilePath();
info.isDir = entry.isDir();
info.size = entry.size();
int row = m_fileInfos.count();
m_fileInfos.append(info);
QModelIndex index = createIndex(row, 0, &m_fileInfos[row]);
if (parent.isValid()) {
insertRow(row, parent);
}
if (info.isDir) {
populateModel(info.path, index);
}
}
}
```
最后,我们可以在可视化控件中使用这个模型,如下所示:
```cpp
QString path = "/path/to/folder";
FileSystemModel *model = new FileSystemModel(this);
model->populateModel(path, QModelIndex());
QTreeView *view = new QTreeView(this);
view->setModel(model);
```
这样就可以在QTreeView控件中显示指定路径下的文件和目录了。当用户单击某个项目时,我们可以根据其索引获取该项目的信息,以便执行相关操作。
相关推荐
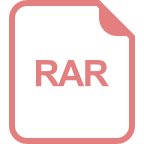
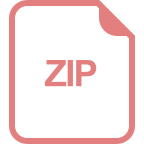
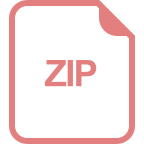














