java输出一个空心圆形
时间: 2024-05-16 15:12:16 浏览: 26
你可以使用以下Java代码输出一个空心圆形:
```
public class Main {
public static void main(String[] args) {
int radius = 5;
for (int i = -radius; i <= radius; i++) {
for (int j = -radius; j <= radius; j++) {
double distance = Math.sqrt(i * i + j * j);
if (distance > radius - 0.5 && distance < radius + 0.5) {
System.out.print("* ");
} else {
System.out.print(" ");
}
}
System.out.println();
}
}
}
```
这段代码会输出一个半径为5的空心圆形。
相关问题
用Java写一个输入边长,输出用*组成的空心圆形
好的,这是一个关于编程的问题,我可以回答。以下是Java程序代码:
```
import java.util.Scanner;
public class HollowCircle {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Please enter the length of the side: ");
int side = input.nextInt();
for (int i = 1; i <= side * 2 - 1; i++) {
for (int j = 1; j <= side * 2 - 1; j++) {
int x = Math.abs(side - i);
int y = Math.abs(side - j);
double distance = Math.sqrt(x * x + y * y);
if (distance > side - 1 && distance < side) {
System.out.print("*");
} else {
System.out.print(" ");
}
}
System.out.println();
}
}
}
```
您可以将此代码复制并粘贴到您的Java开发环境中运行。程序会要求您输入边长,并输出以 * 组成的空心圆形。
Java写一个hollow World
### 回答1:
你好,这是一个简单的 Java 代码,用于输出 "hollow world"。
```
public class HelloWorld {
public static void main(String[] args) {
System.out.println("hollow world");
}
}
```
希望能对你有所帮助。
### 回答2:
Java中可以使用基本的图形库来编写一个空心的世界。下面是一个示例代码:
```java
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
public class HollowWorld extends JFrame {
public HollowWorld() {
setTitle("Hollow World");
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
@Override
public void paint(Graphics g) {
super.paint(g);
g.setColor(Color.BLACK);
int centerX = getWidth() / 2;
int centerY = getHeight() / 2;
int radius = Math.min(getWidth(), getHeight()) / 4;
g.drawOval(centerX - radius, centerY - radius, radius * 2, radius * 2);
}
public static void main(String[] args) {
new HollowWorld();
}
}
```
使用上述代码,首先创建一个JFrame对象,然后调用其paint方法来绘制一个空心的圆形世界。在paint方法中,我们将画笔的颜色设为黑色,并根据窗口大小计算圆心的位置和半径,最后使用drawOval方法画出一个空心圆形。
### 回答3:
Java中使用图形库可以绘制各种图形,包括一个空心的圆。
首先我们需要引入Java的图形库,并创建一个绘图窗口。
```java
import javax.swing.*;
import java.awt.*;
public class HollowWorld extends JPanel {
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
int width = getWidth();
int height = getHeight();
// 绘制一个空心的圆
int radius = Math.min(width, height) / 2 - 10;
int centerX = width / 2;
int centerY = height / 2;
g2d.setStroke(new BasicStroke(3)); // 设置线宽为3
g2d.drawOval(centerX - radius, centerY - radius, radius * 2, radius * 2);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Hollow World");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 400);
frame.add(new HollowWorld());
frame.setVisible(true);
}
}
```
上述代码创建了一个继承自JPanel的类`HollowWorld`,在`paintComponent`方法中绘制了一个空心的圆。在`main`方法中创建一个窗口并将`HollowWorld`添加进去。
运行以上代码,将会弹出一个窗口显示一个空心的圆。
阅读全文
相关推荐
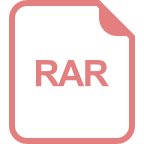
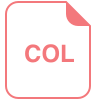
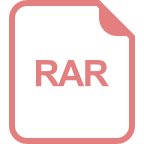
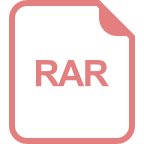
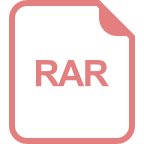
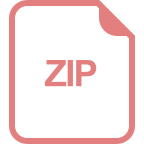