利用A*算法实现八数码难题(九宫重排)的搜索。
时间: 2023-07-20 08:26:05 浏览: 53
好的,我可以帮你解答这个问题。八数码难题又称九宫重排,是一个经典的AI问题,目标是将一个3x3的九宫格中,数字1-8和一个空格按照一定的规则排列成目标状态,如下图所示:
```
1 2 3
4 5 6
7 8
```
A*算法是一种启发式搜索算法,通过估计当前状态到目标状态的距离来选择下一步的搜索方向,以达到更快的搜索速度和更好的搜索效果。在八数码难题中,我们可以用曼哈顿距离来估计当前状态到目标状态的距离。
具体实现步骤如下:
1. 将当前状态和目标状态表示成一个3x3的矩阵。
2. 定义状态节点类,包括当前状态、空格位置、曼哈顿距离、路径长度等信息。
3. 定义启发函数,计算当前状态到目标状态的曼哈顿距离。
4. 定义状态扩展函数,生成当前状态的所有可行子状态。
5. 使用优先队列保存节点,按照f(n)=g(n)+h(n)的顺序进行排序,其中g(n)为当前状态的路径长度,h(n)为当前状态到目标状态的估计距离。
6. 从优先队列中取出f(n)最小的节点,扩展其子状态,并计算子状态的f(n)值,将其加入优先队列中。
7. 重复步骤6,直到找到目标状态或者队列为空。
8. 输出路径,即从初始状态到目标状态的最短路径。
希望这个回答能够帮到你,如果你还有其他问题,可以继续问我哦。
相关问题
利用A*算法实现八数码难题(九宫重排)的搜索
八数码难题是一种经典的搜索问题,使用A*算法可以高效地解决。以下是A*算法解决八数码难题的步骤:
1. 定义状态表示:将八数码难题的每个状态表示为一个3x3的矩阵,其中空格用0表示,其他数字用1~8表示。
2. 定义状态转移操作:定义状态转移操作为将空格与相邻的数字交换位置,注意边界处理。
3. 定义启发函数:启发函数用于评估当前状态到目标状态的距离,常用的启发函数有曼哈顿距离和不在位数字数目。曼哈顿距离定义为每个数字到目标位置的曼哈顿距离之和,不在位数字数目定义为当前状态和目标状态中数字不同的数量。
4. 定义状态集合和搜索队列:状态集合用于存储已经访问过的状态,搜索队列用于存储待搜索状态。
5. 初始化搜索队列和状态集合:将初始状态加入搜索队列,将状态集合清空。
6. 进入循环:从搜索队列中取出f(n)值最小的状态进行扩展,如果该状态已经被访问过,则跳过。否则,将该状态加入状态集合,并按照状态转移操作生成其所有邻居状态。对于每个邻居状态,计算其f(n)值并将其加入搜索队列。
7. 判断是否达到目标状态:如果当前状态为目标状态,则搜索结束。
8. 输出结果:输出从初始状态到目标状态的路径。
以下是Python代码实现A*算法解决八数码难题:
```python
import heapq
# 定义状态转移操作
def move(state, x, y, nx, ny):
new_state = [row[:] for row in state]
new_state[x][y], new_state[nx][ny] = new_state[nx][ny], new_state[x][y]
return new_state
# 定义启发函数
def h(state, goal):
return sum([abs(state[i][j] // 3 - goal[i][j] // 3) + abs(state[i][j] % 3 - goal[i][j] % 3) for i in range(3) for j in range(3)])
# 初始化搜索队列和状态集合
start_state = [[2, 8, 3], [1, 6, 4], [7, 0, 5]]
goal_state = [[1, 2, 3], [8, 0, 4], [7, 6, 5]]
g = {str(start_state): 0}
f = {str(start_state): h(start_state, goal_state)}
queue = []
heapq.heappush(queue, (f[str(start_state)], start_state))
# 进入循环
while queue:
curr_f, curr_state = heapq.heappop(queue)
if curr_state == goal_state:
break
for i in range(3):
for j in range(3):
if curr_state[i][j] == 0:
x, y = i, j
for nx, ny in [(x-1, y), (x+1, y), (x, y-1), (x, y+1)]:
if 0 <= nx < 3 and 0 <= ny < 3:
new_state = move(curr_state, x, y, nx, ny)
new_g = g[str(curr_state)] + 1
if str(new_state) not in g or new_g < g[str(new_state)]:
g[str(new_state)] = new_g
f[str(new_state)] = new_g + h(new_state, goal_state)
heapq.heappush(queue, (f[str(new_state)], new_state))
# 输出结果
path = [curr_state]
while str(curr_state) in g:
curr_state = min([state for state in [move(path[0], x, y, nx, ny) for nx, ny in [(y, x-1), (y, x+1), (y-1, x), (y+1, x)] if 0 <= nx < 3 and 0 <= ny < 3] if str(state) in g], key=lambda state: g[str(state)])
path.insert(0, curr_state)
for state in path:
print(state)
print("Total number of moves:", len(path)-1)
```
利用A*算法实现八数码难题(九宫重排)的搜索c++代码
下面是一个用C++实现的八数码问题A*算法搜索代码,其中使用了曼哈顿距离作为估价函数:
```c++
#include <iostream>
#include <queue>
#include <vector>
#include <map>
using namespace std;
// 数码状态结构体
struct Node {
int state[3][3];
int x, y; // 空格的位置
int f, g, h; // f=g+h
Node *father; // 父状态指针
// 构造函数
Node(int s[3][3], int x, int y, int g, Node *father) {
for (int i = 0; i < 3; i++)
for (int j = 0; j < 3; j++)
state[i][j] = s[i][j];
this->x = x;
this->y = y;
this->g = g;
this->h = 0;
this->father = father;
calcH(); // 计算估价函数值
calcF(); // 计算f值
}
// 拷贝构造函数
Node(const Node &rhs) {
for (int i = 0; i < 3; i++)
for (int j = 0; j < 3; j++)
state[i][j] = rhs.state[i][j];
this->x = rhs.x;
this->y = rhs.y;
this->g = rhs.g;
this->h = rhs.h;
this->father = rhs.father;
calcF();
}
// 计算估价函数值
void calcH() {
int cnt = 0;
for (int i = 0; i < 3; i++)
for (int j = 0; j < 3; j++) {
if (state[i][j] == 0) continue;
int x = (state[i][j] - 1) / 3;
int y = (state[i][j] - 1) % 3;
cnt += abs(x - i) + abs(y - j);
}
this->h = cnt;
}
// 计算f值
void calcF() {
this->f = this->g + this->h;
}
};
// 重载小于运算符,用于优先队列排序
bool operator < (Node a, Node b) {
return a.f > b.f;
}
// 判断状态是否合法
bool isLegal(Node *p) {
int cnt = 0;
for (int i = 0; i < 9; i++) {
int x1 = i / 3, y1 = i % 3;
if (p->state[x1][y1] == 0) continue;
for (int j = i + 1; j < 9; j++) {
int x2 = j / 3, y2 = j % 3;
if (p->state[x2][y2] == 0) continue;
if (p->state[x1][y1] > p->state[x2][y2]) cnt++;
}
}
return cnt % 2 == 0;
}
// 判断状态是否为目标状态
bool isGoal(Node *p) {
int cnt = 1;
for (int i = 0; i < 3; i++)
for (int j = 0; j < 3; j++) {
if (cnt == 9) return true;
if (p->state[i][j] != cnt++) return false;
}
return true;
}
// 打印状态
void printState(Node *p) {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << p->state[i][j] << " ";
}
cout << endl;
}
cout << endl;
}
// A*算法搜索
void AStar(Node *start) {
priority_queue<Node> open; // open表,按f值从小到大排序
map<string, bool> closed; // closed表,用于判重
open.push(*start);
closed[string((char *)start->state, 9)] = true;
while (!open.empty()) {
Node cur = open.top();
open.pop();
if (isGoal(&cur)) {
cout << "Find the goal state:" << endl;
printState(&cur);
return;
}
// 生成下一层状态
int dx[] = {0, 0, -1, 1};
int dy[] = {-1, 1, 0, 0};
for (int i = 0; i < 4; i++) {
int nx = cur.x + dx[i];
int ny = cur.y + dy[i];
if (nx < 0 || nx >= 3 || ny < 0 || ny >= 3) continue;
Node next(cur);
swap(next.state[cur.x][cur.y], next.state[nx][ny]);
next.x = nx;
next.y = ny;
next.g++;
next.father = &cur;
// 判断状态是否合法和是否已经访问过
if (isLegal(&next) && !closed[string((char *)next.state, 9)]) {
open.push(next);
closed[string((char *)next.state, 9)] = true;
}
}
}
cout << "Can't find the goal state!" << endl;
}
int main() {
// 初始状态
int startState[3][3] = {
{2, 8, 3},
{1, 6, 4},
{7, 0, 5}
};
Node *start = new Node(startState, 1, 2, 0, NULL);
AStar(start);
return 0;
}
```
注意,在该代码中,使用了一个`map`容器来进行状态的判重。此外,为了使用`map`容器,还需要重载`Node`结构体的`<`运算符和构造函数。
相关推荐
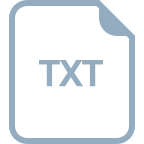
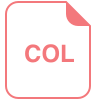
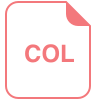
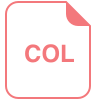
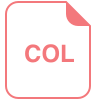
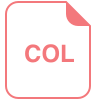







