linux c++ 串口通信封装代码
时间: 2023-10-25 18:06:57 浏览: 160
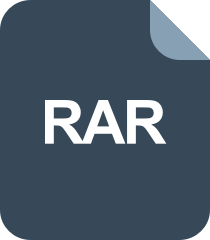
C++串口通信代码
你好,以下是一个基本的串口通信封装代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#include <string.h>
int set_port(int fd, int baud_rate) {
struct termios options;
tcgetattr(fd, &options);
switch (baud_rate) {
case 9600:
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
break;
case 19200:
cfsetispeed(&options, B19200);
cfsetospeed(&options, B19200);
break;
case 38400:
cfsetispeed(&options, B38400);
cfsetospeed(&options, B38400);
break;
case 57600:
cfsetispeed(&options, B57600);
cfsetospeed(&options, B57600);
break;
case 115200:
cfsetispeed(&options, B115200);
cfsetospeed(&options, B115200);
break;
default:
return -1;
}
options.c_cflag |= CLOCAL;
options.c_cflag |= CREAD;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cc[VMIN] = 1;
options.c_cc[VTIME] = 0;
tcflush(fd, TCIFLUSH);
if (tcsetattr(fd, TCSANOW, &options) != 0) {
return -1;
}
return 0;
}
int open_port(char *port_name) {
int fd;
fd = open(port_name, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
return -1;
}
fcntl(fd, F_SETFL, 0);
return fd;
}
int serial_send(int fd, char *data) {
int len = strlen(data);
int res = write(fd, data, len);
if (res != len) {
return -1;
}
return 0;
}
int serial_receive(int fd, char *buffer, int buffer_size) {
memset(buffer, 0, buffer_size);
int res = read(fd, buffer, buffer_size - 1);
if (res == -1) {
return -1;
}
buffer[res] = 0;
return res;
}
int main(int argc, char *argv[]) {
int fd;
char buffer[256];
fd = open_port("/dev/ttyS0");
if (fd == -1) {
printf("Failed to open serial port!\n");
exit(1);
}
set_port(fd, 9600);
while (1) {
printf("Please enter data to send (q to quit):\n");
fgets(buffer, sizeof(buffer), stdin);
if (buffer[0] == 'q') {
break;
}
serial_send(fd, buffer);
serial_receive(fd, buffer, sizeof(buffer));
printf("%s\n", buffer);
}
close(fd);
return 0;
}
```
希望对你有帮助!
阅读全文
相关推荐

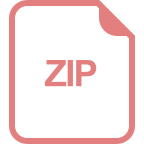
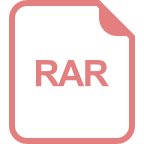
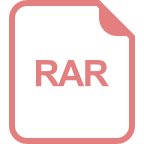
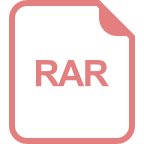
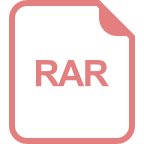
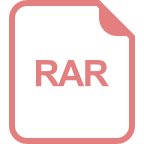
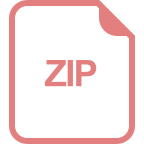
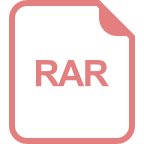
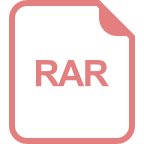
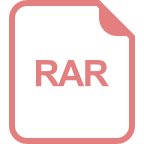
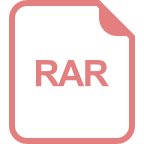
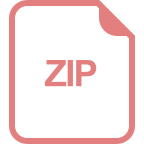
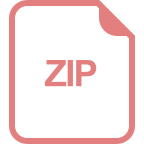
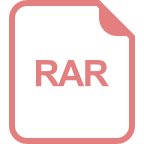
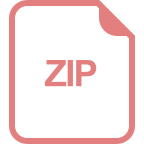