修改HTTP401返回消息
时间: 2024-09-10 14:10:51 浏览: 65
当服务器遇到未授权的情况,并发送HTTP 401 Unauthorized状态码响应给客户端时,通常会包含一个简单的错误信息以及一个`WWW-Authenticate`头部,指示需要提供适当的认证凭证才能访问资源。如果你想要修改这个返回的消息,特别是在Web应用或API服务中,你可以自定义返回体内容,包括但不限于:
1. 更友好的错误提示:例如从"Unauthorized"改为"用户名或密码无效"。
2. 提供额外的信息:如错误原因、重试建议等。
以下是一个简单的示例(假设使用Node.js和Express框架):
```javascript
app.use((req, res, next) => {
if (req.unauthorized) { // 检查请求是否未经身份验证
let message = '您的凭据无效,请检查并重试。';
res.status(401)
.header('WWW-Authenticate', 'Bearer realm="Your Realm"')
.json({ error: message, details: 'Please provide valid credentials.' });
} else {
next(); // 如果已经授权,则继续处理请求
}
});
```
在这个例子中,`res.json()`用于定制返回的JSON对象,包含了自定义的错误信息和详细说明。
相关问题
jwt 401 手动 抛出 401
要手动抛出401错误,您可以使用HTTP响应状态码和相应的错误消息。在JWT验证失败时,您可以返回一个包含401状态码的响应,并提供一个错误消息,告诉客户端身份验证失败。
以下是一个示例代码片段,展示了如何在Python Flask框架中手动抛出401错误:
```python
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/protected-resource')
def protected_resource():
# 在这里进行JWT验证
# 如果验证失败,手动抛出401错误
if not verify_jwt_token():
response = jsonify({'error': 'JWT authentication failed'})
response.status_code = 401
return response
# 在这里处理受保护资源的逻辑
return jsonify({'message': 'You have access to protected resource'})
def verify_jwt_token():
# 在这里进行JWT验证逻辑
# 返回True表示验证通过,返回False表示验证失败
pass
if __name__ == '__main__':
app.run()
```
在上述示例中,当`verify_jwt_token()`函数返回False时,我们手动创建一个包含错误消息的JSON响应,并将状态码设置为401。这样,客户端就知道身份验证失败并可以采取适当的措施。
请注意,这只是一个示例,并且可以根据您的具体需求进行修改。
spring security修改返回值
如果你想要修改Spring Security的返回值,可以通过自定义异常处理器来实现。首先,你需要创建一个实现了`AuthenticationEntryPoint`接口的异常处理器类,例如:
```java
@Component
public class CustomAuthenticationEntryPoint implements AuthenticationEntryPoint {
@Override
public void commence(HttpServletRequest request, HttpServletResponse response, AuthenticationException authException) throws IOException, ServletException {
response.setStatus(HttpStatus.UNAUTHORIZED.value());
response.setContentType(MediaType.APPLICATION_JSON_VALUE);
response.getWriter().write(new ObjectMapper().writeValueAsString("Custom Unauthorized Message"));
}
}
```
在这个异常处理器中,我们可以通过`response`对象来修改返回的HTTP状态码、响应头和响应体。这里我们将状态码设置为401,响应类型设置为JSON格式,响应体设置为一个自定义的未授权消息。
然后,我们需要在Spring Security的配置文件中将这个异常处理器添加进去,例如:
```java
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private CustomAuthenticationEntryPoint customAuthenticationEntryPoint;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.exceptionHandling()
.authenticationEntryPoint(customAuthenticationEntryPoint)
.and()
.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
```
在这个配置文件中,我们将自定义的异常处理器添加到了`exceptionHandling()`方法中,这样当用户未经授权访问受保护的资源时,就会由这个异常处理器来处理返回的结果。
阅读全文
相关推荐
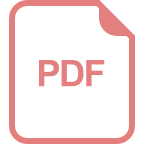
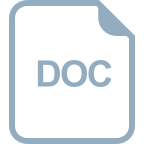
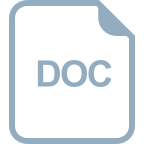
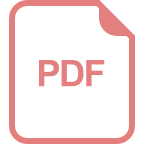
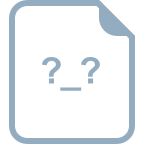
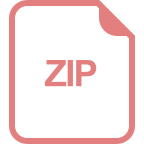
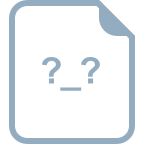
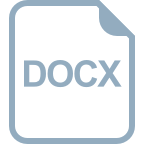
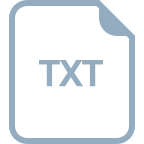
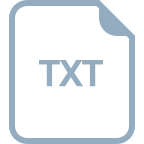
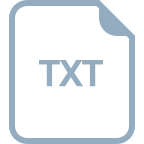
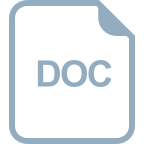
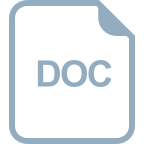
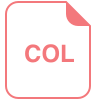
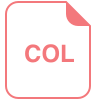
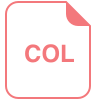