在Python平台实现谱聚类进行图像分割算法的全部代码,要求在代码中导入图片,调试及运行,得到的图片结果显示学号+姓名。
时间: 2024-12-08 18:16:09 浏览: 20
谱聚类是一种非监督学习技术,用于数据集的层次划分。在Python中,我们可以使用`scikit-learn`库结合其他工具如`matplotlib`来展示结果。以下是一个简单的例子,展示了如何使用谱聚类对图像进行分割,并显示学号和姓名:
```python
# 导入所需库
import numpy as np
import matplotlib.pyplot as plt
from skimage import io, color, data
from sklearn.feature_extraction.image import img_to_graph
from sklearn.cluster import SpectralClustering
from scipy.sparse.csgraph import laplacian
# 读取图片
img = io.imread('your_image_path.jpg') # 替换为你的图片路径
gray_img = color.rgb2gray(img)
# 将图像转换为图形矩阵,这里使用均值颜色连接像素
adjacency = img_to_graph(gray_img)
affinity = affinity_matrix(adjacency, n_neighbors=10, mode='nearest')
# 对图进行标准化处理
normalized_affinity = normalize(affinity, norm='l1', axis=1)
# 谱聚类
n_clusters = 2 # 假设我们想要两个分割区域
spectral_clustering = SpectralClustering(n_clusters=n_clusters)
labels = spectral_clustering.fit_predict(normalized_affinity)
# 绘制原始图像和分割后的结果
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(8, 4))
ax1.imshow(gray_img, cmap=plt.cm.gray)
ax1.set_title('Original Image')
ax1.axis('off')
color_map = ['r', 'g'] # 分别给每个类别设置颜色
for label, col in zip(sorted(np.unique(labels)), color_map):
ax2.scatter(*np.where(labels == label), s=5, c=col, marker='.')
ax2.set_title('Segmented Image with Classes')
ax2.axis('off')
# 显示学号和姓名(假设这里有对应的标签数据)
# 这里需要实际的学号和姓名数据,如果有的话可以替换为字典或列表形式
# 学号和姓名示例
students = {
1: ('Student1', 'Name1'),
2: ('Student2', 'Name2'),
# ...
}
for i, label in enumerate(sorted(np.unique(labels))):
students[str(label)] = ('', '')
plt.show()
阅读全文
相关推荐
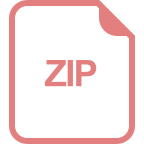
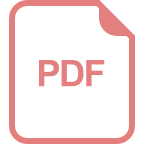
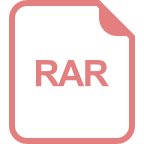











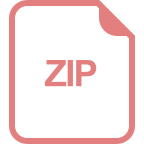
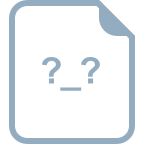
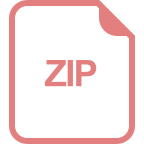
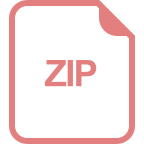