写两个简单的Django渲染模板的例子
时间: 2024-11-03 18:20:23 浏览: 14
在Django中,模板是用来显示数据给用户的HTML结构。你可以通过视图函数将数据传递到模板,然后模板负责呈现。这里有两个简单的例子:
**1. 显示单个变量的例子 (views.py)**
```python
from django.shortcuts import render
def simple_template(request):
name = "John Doe" # 假设这是从数据库获取的数据
return render(request, 'example.html', {'name': name})
```
在这个例子中,`render`函数接收请求、模板名('example.html')以及一个字典{'name': 'John Doe'},其中'name'是模板中可以使用的变量。
**2. 使用循环渲染列表的例子 (views.py)**
```python
from django.shortcuts import render
from .models import MyModel # 假设MyModel是一个包含标题和内容的模型
def list_templates(request):
objects = MyModel.objects.all() # 获取所有模型实例
context = {'items': objects}
return render(request, 'list_example.html', context)
```
在这个例子中,视图获取`MyModel`的所有对象,并将其作为一个列表存储在'dict_items'中,然后传递给模板`list_example.html`。
**对应的模板文件 (example.html 或 list_example.html)**
```html
<!-- example.html -->
<html>
<head>
<title>{{ name }}</title>
</head>
<body>
Hello, {{ name }}!
</body>
</html>
<!-- list_example.html -->
<html>
<head>
<title>Items List</title>
</head>
<body>
{% for item in items %}
<p>Title: {{ item.title }}, Content: {{ item.content }}</p>
{% endfor %}
</body>
</html>
```
在模板中,我们使用了Django模板语言(如`{{ variable_name }}`),它会根据视图传递的上下文数据动态填充内容。
阅读全文
相关推荐
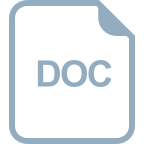
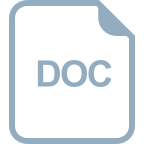
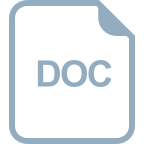
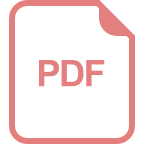
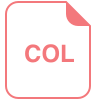
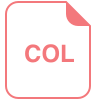
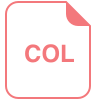
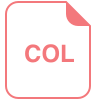
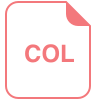
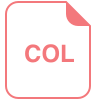
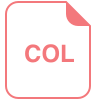
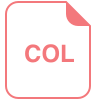
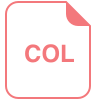

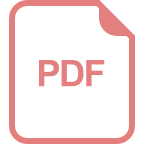
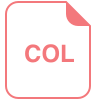
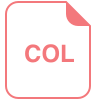
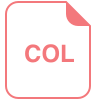
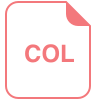