C++ vector 如何插入数据
时间: 2024-09-10 10:29:05 浏览: 47
在C++中,`vector`是标准模板库(STL)中的一个动态数组容器,它允许在序列的任何位置快速插入新元素。以下是几种在`vector`中插入数据的方法:
1. 使用`push_back()`函数在`vector`的末尾插入一个元素。这个函数会自动将元素添加到`vector`的末尾,并且如果需要,`vector`会进行扩容操作。
```cpp
std::vector<int> vec;
vec.push_back(10); // 在vector末尾插入元素10
```
2. 使用`insert()`函数在`vector`的指定位置插入一个或多个相同的元素,或者插入一个范围内的元素。
- 插入单个元素:
```cpp
vec.insert(vec.begin() + 5, 20); // 在vector的第5个位置(从0开始计数)插入元素20
```
- 插入多个相同的元素:
```cpp
vec.insert(vec.begin() + 5, 3, 30); // 在vector的第5个位置插入3个元素30
```
- 插入另一个容器中的所有元素:
```cpp
std::vector<int> anotherVec = {40, 50, 60};
vec.insert(vec.begin() + 5, anotherVec.begin(), anotherVec.end()); // 在vector的第5个位置插入anotherVec中的所有元素
```
3. 使用`emplace`函数在`vector`的指定位置直接构造一个新元素。这个方法的优势在于它可以直接在`vector`内部构造对象,避免了不必要的拷贝或移动操作。
```cpp
vec.emplace(vec.begin() + 5, 70); // 在vector的第5个位置直接构造元素70
```
请注意,使用`insert()`和`emplace()`函数插入元素时,如果指定的位置超出了当前`vector`的大小,新插入的元素将会被添加到`vector`末尾。
阅读全文
相关推荐
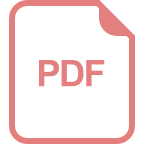
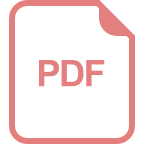
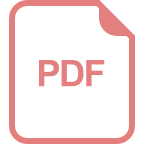
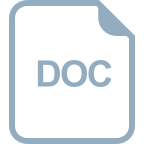
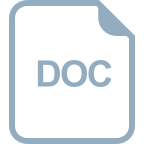
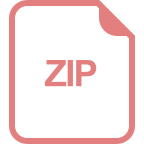
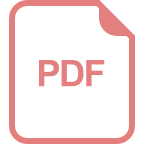
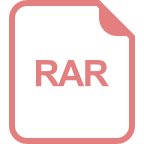
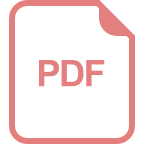
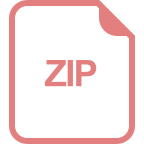
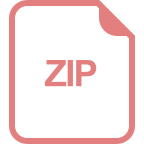
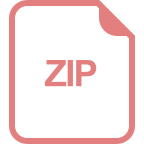
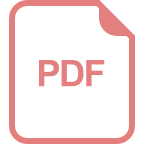
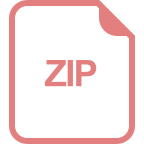
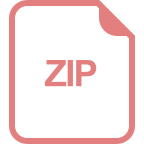
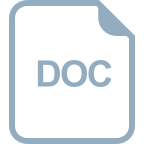
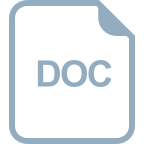
