在C语言中,有一个1.txt的文件,里面有数据,把文件里面的数据读出来写到2.txt文件里,即把1.txt的文件复制 到2.txt文件里面
时间: 2024-12-16 16:27:31 浏览: 11
在C语言中,你可以使用标准库函数`fopen()`, `fclose()`, `fread()`, 和 `fwrite()` 来实现文件的复制操作。这里是一个简单的示例:
```c
#include <stdio.h> // 引入文件I/O头文件
int main() {
FILE *input_file, *output_file; // 定义输入和输出文件指针
// 打开输入文件并检查是否成功
input_file = fopen("1.txt", "r");
if (input_file == NULL) {
perror("Error opening input file");
return 1;
}
// 打开输出文件,如果不存在则创建
output_file = fopen("2.txt", "w+");
if (output_file == NULL) {
perror("Error creating output file");
fclose(input_file); // 关闭已打开的输入文件
return 1;
}
// 读取输入文件的内容并写入输出文件
char buffer[BUFSIZ]; // 用于临时存储读取的数据
size_t bytes_read;
while ((bytes_read = fread(buffer, sizeof(char), BUFSIZ, input_file)) > 0) {
fwrite(buffer, sizeof(char), bytes_read, output_file);
}
// 检查是否有错误发生
if (ferror(input_file) || ferror(output_file)) {
perror("Error reading or writing to the files");
fclose(input_file);
fclose(output_file);
return 1;
}
// 文件操作完成后关闭它们
fclose(input_file);
fclose(output_file);
printf("File copied successfully.\n");
return 0;
}
```
在这个例子中,程序首先尝试打开名为"1.txt"的文件作为输入,并以写模式("w+")打开"2.txt"文件作为输出。然后它会逐块读取"1.txt"的内容,将内容写入"2.txt"直到所有数据都读完。
阅读全文
相关推荐
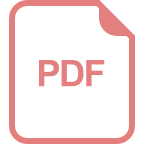
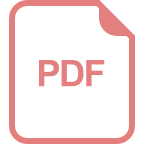
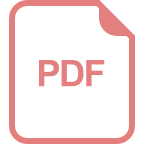
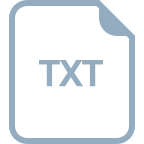
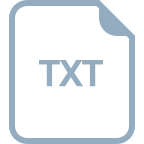
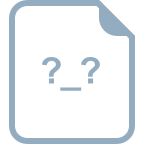
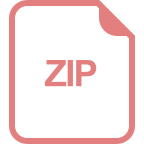
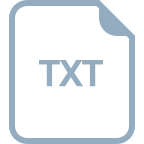
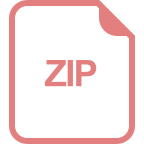
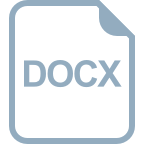
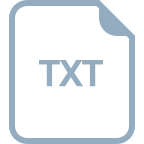
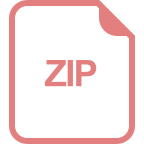
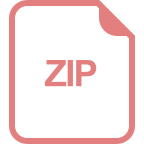
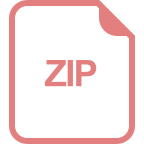
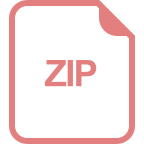