请帮我用c#写一个代码,1-1/2+1/3-1/4+1/5-1/6+…+1/(n-1)-1/n求n=100的时候的和
时间: 2024-11-27 09:18:50 浏览: 12
在C#中,你可以使用循环和条件语句来编写这样的程序。这是一个简单的实现,用于计算从1到n的所有分数之和,其中正负交替:
```csharp
using System;
class Program {
static void Main(string[] args) {
int n = 100;
double sum = 0.0;
bool isPositive = true;
for (int i = 1; i <= n; i++) {
if (isPositive) {
sum += 1.0 / i;
} else {
sum -= 1.0 / i;
}
// 每隔两个数改变正负
isPositive = !isPositive;
}
Console.WriteLine($"The sum of the alternating fractions from 1 to {n} is: {sum}");
}
}
```
这个代码会打印出当n等于100时,1到100所有分数加减后的总和。
阅读全文
相关推荐
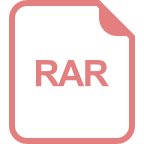
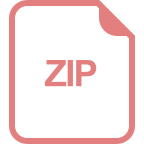
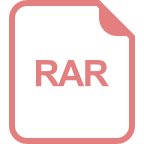
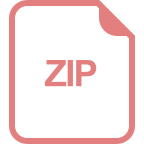
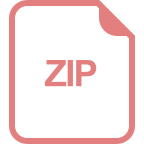
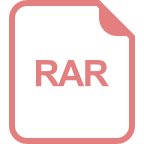
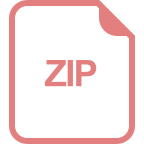
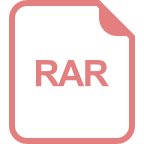
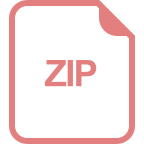
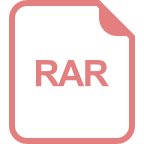
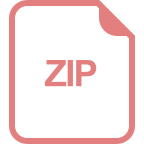
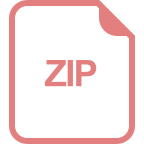
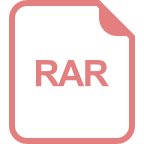
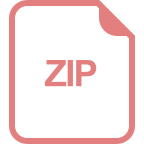
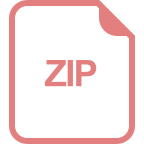
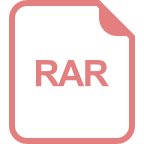
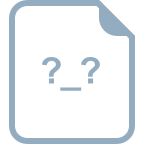