常用类的封装,文件上传类,判断路径是否可用,获取文件信息,检查文件大小,检查文件后缀,移动文件至指定目录的方法,显示错误信息的方法PHP
时间: 2024-06-13 14:08:20 浏览: 37
以下是一个常用的PHP文件上传类的封装,其中包含了判断路径是否可用、获取文件信息、检查文件大小、检查文件后缀、移动文件至指定目录的方法以及显示错误信息的方法:
```php
class FileUploader {
private $allowedExtensions = array();
private $sizeLimit = 10485760;
private $file;
function __construct(array $allowedExtensions = array(), $sizeLimit = 10485760){
$allowedExtensions = array_map("strtolower", $allowedExtensions);
$this->allowedExtensions = $allowedExtensions;
$this->sizeLimit = $sizeLimit;
$this->checkServerSettings();
if (isset($_FILES['file'])) {
$this->file = new File($_FILES['file']);
}
}
private function checkServerSettings(){
$postSize = ini_get('post_max_size');
$unit = strtoupper(substr($postSize, -1));
$postSize = (int)substr($postSize, 0, -1);
if ($unit == 'G') {
$postSize *= 1024 * 1024 * 1024;
} else if ($unit == 'M') {
$postSize *= 1024 * 1024;
} else if ($unit == 'K') {
$postSize *= 1024;
}
if ($postSize < $this->sizeLimit) {
$this->sizeLimit = $postSize;
}
}
function upload($uploadDirectory){
if (!is_writable($uploadDirectory)) {
return "上传目录不存在或不可写";
}
if (!$this->file) {
return "没有文件被上传";
}
$size = $this->file->size();
if ($size == 0) {
return "文件大小为0";
}
if ($size > $this->sizeLimit) {
return "文件大小超过限制";
}
$pathinfo = pathinfo($this->file->name());
$filename = $pathinfo['filename'];
$ext = $pathinfo['extension'];
if ($this->allowedExtensions && !in_array(strtolower($ext), $this->allowedExtensions)) {
return "文件类型不被允许";
}
$filename = md5(uniqid($filename));
$filename .= '.'.$ext;
$filePath = $uploadDirectory.'/'.$filename;
if ($this->file->save($filePath)) {
return $filename;
} else {
return "文件上传失败";
}
}
}
class File {
private $file;
function __construct($file){
$this->file = $file;
}
function name(){
return $this->file['name'];
}
function size(){
return $this->file['size'];
}
function save($path){
return move_uploaded_file($this->file['tmp_name'], $path);
}
}
```
阅读全文
相关推荐
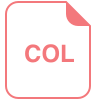
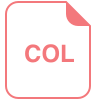
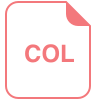
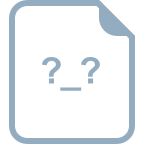
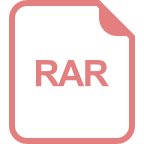
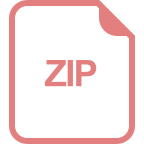
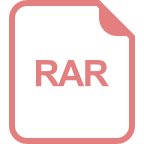
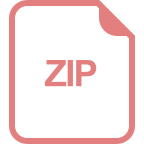
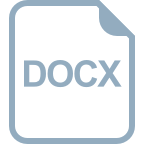
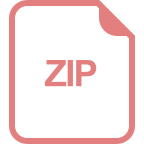
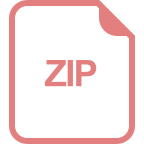
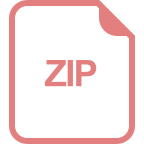