Java 读取Word书签并替换书签内容
时间: 2023-09-14 20:03:15 浏览: 82
你可以使用 Apache POI 库来读取 Word 文档并操作书签。以下是一个 Java 示例代码,可以读取 Word 文档中的指定书签,并将其内容替换为指定的文本:
```java
import java.io.FileInputStream;
import java.io.FileOutputStream;
import org.apache.poi.openxml4j.exceptions.InvalidFormatException;
import org.apache.poi.poifs.filesystem.POIFSFileSystem;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.apache.xmlbeans.XmlCursor;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTBookmark;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTMarkupRange;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTText;
public class WordBookmarkReplace {
public static void main(String[] args) throws Exception {
String filePath = "path/to/word/document.docx";
String bookmarkName = "bookmark1";
String replacementText = "replacement text";
// Load the Word document
POIFSFileSystem fs = new POIFSFileSystem(new FileInputStream(filePath));
XWPFDocument doc = new XWPFDocument(fs);
// Find the bookmark by name
CTBookmark bookmark = findBookmarkByName(doc, bookmarkName);
if (bookmark == null) {
throw new IllegalArgumentException("Bookmark not found: " + bookmarkName);
}
// Replace the bookmark content with the replacement text
CTMarkupRange range = bookmark.getDomNode().getFirstChild().getDomNode().getFirstChild();
XmlCursor cursor = range.newCursor();
XWPFParagraph p = doc.getParagraphArray(cursor.getBookmarkId());
XWPFRun r = p.getRuns().get(0);
r.setText(replacementText, 0);
// Save the modified document
FileOutputStream out = new FileOutputStream(filePath);
doc.write(out);
out.close();
doc.close();
}
private static CTBookmark findBookmarkByName(XWPFDocument doc, String name) {
for (CTBookmark bookmark : doc.getDocument().getBody().getSdtArray()) {
if (bookmark.getName().equals(name)) {
return bookmark;
}
}
return null;
}
}
```
在这个示例中,我们首先加载 Word 文档,然后使用 `findBookmarkByName` 方法查找指定名称的书签。如果找到了书签,我们就可以使用其范围信息找到书签所在的段落和文本,并将其替换为指定的文本。最后,我们将修改后的文档保存回原始文件。
相关推荐
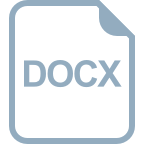














