#include <iostream> #include <string> using namespace std; typedef string ElemType; typedef struct BitNode { ElemType data; struct BitNode *lchild, *rchild; } BitNode, *BiTree; void CreateBiTree(BiTree &t) { string ch; cin >> ch; if (ch[0] == '#') { t = NULL; } else { t = new BitNode; t->data = ch; CreateBiTree(t->lchild); CreateBiTree(t->rchild); } } void InOrderTraverse(BiTree t) { if (t) { string op = t->data; if (op[0] < '0' || op[0] > '9') cout << '('; InOrderTraverse(t->lchild); cout << t->data; InOrderTraverse(t->rchild); if (op[0] < '0' || op[0] > '9') cout << ')'; } } int main() { BiTree tree = new BitNode; string ch; while (cin >> ch) { tree->data = ch; CreateBiTree(tree->lchild); CreateBiTree(tree->rchild); InOrderTraverse(tree); printf("\n"); } return 0; }换一个算法实现这个代码
时间: 2024-03-14 19:46:12 浏览: 52
这段代码实现了对二叉树的创建和中序遍历,并且输出的遍历结果包含了括号,用于表示运算符的优先级。如果需要换一个算法实现这个代码,可以使用栈来实现二叉树的创建和中序遍历。
具体实现方法如下:
1. 定义一个栈,用于存放节点;
2. 从输入中读取每一个元素,然后创建一个节点;
3. 如果当前元素为操作数,则将其压入栈中;
4. 如果当前元素为操作符,则将其作为根节点,将栈顶的两个节点分别作为左右子节点,并将根节点压入栈中;
5. 当所有元素都处理完毕后,栈中剩余的节点即为二叉树的根节点。
具体代码实现如下:
```C++
#include <iostream>
#include <string>
#include <stack>
using namespace std;
typedef string ElemType;
typedef struct BitNode {
ElemType data;
struct BitNode* lchild, * rchild;
} BitNode, * BiTree;
void CreateBiTree(BiTree& t) {
stack<BitNode*> s;
BitNode* p, * q;
string ch;
cin >> ch;
while (ch != "#") {
p = new BitNode;
p->data = ch;
p->lchild = p->rchild = NULL;
if (!t) {
t = p;
}
if (!s.empty()) {
q = s.top();
if (!q->lchild) {
q->lchild = p;
}
else {
q->rchild = p;
s.pop();
}
}
if (ch[0] < '0' || ch[0] > '9') {
s.push(p);
}
cin >> ch;
}
}
void InOrderTraverse(BiTree t) {
if (t) {
string op = t->data;
if (op[0] < '0' || op[0] > '9') {
cout << '(';
}
InOrderTraverse(t->lchild);
cout << t->data;
InOrderTraverse(t->rchild);
if (op[0] < '0' || op[0] > '9') {
cout << ')';
}
}
}
int main() {
BiTree tree = NULL;
string ch;
while (cin >> ch) {
CreateBiTree(tree);
InOrderTraverse(tree);
printf("\n");
tree = NULL;
}
return 0;
}
```
阅读全文
相关推荐
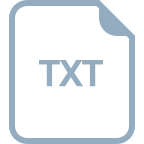
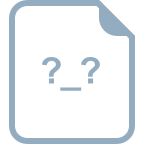















