Unity背包道具如何分帧加载 具体的代码怎么写
时间: 2024-09-16 07:02:11 浏览: 48
在Unity游戏开发中,为了优化性能并避免一次性加载大量道具导致的游戏卡顿,通常会采用分帧(或者说按需)加载的方式。对于背包道具,我们可以利用Unity的资源管理器和C#脚本来实现分帧加载。
首先,你需要在Unity编辑器中将道具预设文件(如Prefab)打包成一种按需加载的形式,例如Resource Provider。这可以通过Unity内置的“Create Prefab Asset Bundle”功能来完成:
1. 将所有需要分帧加载的道具Prefab拖放到Asset Bundle里。
2. 设置每个Prefab的“Streaming”属性为“Required”,以便它们会在首次访问时加载。
然后,在C#代码中,你可以创建一个Resource Provider脚本,通过`UnityEngine.ResourceManager`来加载和卸载道具:
```csharp
using UnityEngine;
using UnityEngine.ResourceManagement;
public class BackpackItemLoader : MonoBehaviour
{
public string assetBundleName;
private AsyncOperation activeLoadOp;
public void LoadItem(string itemName)
{
if (activeLoadOp != null && !activeLoadOp.isDone)
return; // 如果还有其他加载操作中,先等待
activeLoadOp = Resources.LoadAsync(assetBundleName, typeof(UnityEngine.Object));
// 加载完成后回调函数
activeLoadOp.RegisterCallback(onLoaded);
// 当前正在加载,设置道具为null,直到加载完成
_currentItem = null;
}
private void onLoaded(AsyncOperation operation)
{
if (!operation.isDone)
Debug.LogError("Failed to load item!");
object bundleContent = operation.asset;
if (bundleContent is GameObject prefab)
{
Instantiate(prefab); // 根据需要放置到背包或其他位置
_currentItem = prefab.GetComponent<ItemData>(); // 获取道具组件,并设置给当前道具引用
}
else
{
Debug.LogError($"Unexpected content type: {bundleContent.GetType()}");
}
// 清理loadOp
activeLoadOp = null;
}
[HideInInspector] public GameObject currentItem; // 道具实例
}
```
在这个例子中,`LoadItem`方法会异步加载指定名称的道具。当道具加载完毕后,它会被实例化并赋值给`currentItem`字段。如果当前有其他加载请求,则忽略新的请求,直到当前加载任务完成。
阅读全文
相关推荐
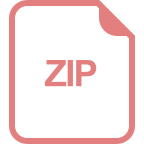
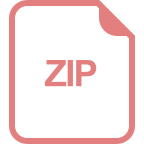
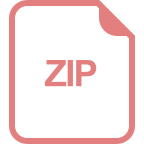

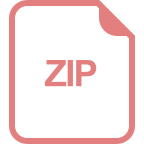
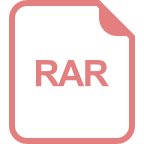
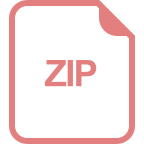
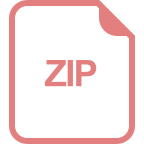
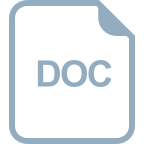
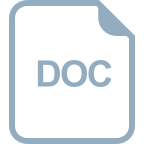
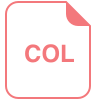
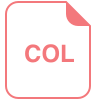
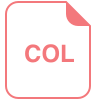

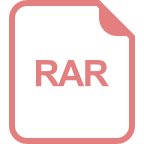
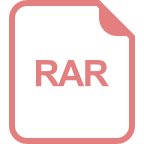
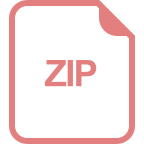
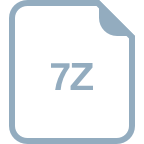