QTC++ windows系统的宏
时间: 2024-06-18 07:02:52 浏览: 239
QTC++是一款跨平台的C++集成开发环境,它支持Windows系统,同时也提供了一些系统相关的宏,方便在Windows系统下进行开发。以下是一些常用的Windows系统相关的宏:
1. WIN32:表示当前编译环境是Windows系统,可以用来判断当前代码是否在Windows下编译。
2. UNICODE:表示当前项目使用Unicode编码。
3. _UNICODE:与UNICODE宏类似,也表示当前项目使用Unicode编码。
4. _WIN32_WINNT:定义Windows操作系统的版本号。
5. _MSC_VER:表示当前使用的Microsoft Visual C++的版本号。
6. __stdcall:定义函数调用方式为stdcall。
7. __declspec:用来声明函数和变量的属性,比如__declspec(dllimport)可以用来导入动态链接库中的函数和变量。
8. _T():用来将字符串转换为TCHAR类型,适配Unicode和ANSI两种编码方式。
相关问题
c++ 将base64 转为图像 并创建一个windows窗口展示出来 不使用qt
要将base64编码的字符串转换为图像并在Windows窗口中展示出来,我们需要进行以下步骤:
1. 将base64编码的字符串解码为二进制数据。
2. 将二进制数据写入到一个文件中,可以使用C++的标准文件流完成。
3. Windows窗口中使用GDI+库加载图像并展示出来。
以下是一个示例代码,演示如何将base64编码的字符串转换为图像并在Windows窗口中展示出来。请注意,此代码使用GDI+库来处理图像。
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <windows.h>
#include <gdiplus.h>
#include <openssl/bio.h>
#include <openssl/evp.h>
#pragma comment(lib, "gdiplus.lib")
#pragma comment(lib, "crypt32.lib")
using namespace Gdiplus;
using namespace std;
// 解码 base64 编码的字符串
string base64_decode(const string& encoded_string) {
BIO *bio, *b64;
char decoded_buffer[encoded_string.size()];
memset(decoded_buffer, 0, sizeof(decoded_buffer));
bio = BIO_new_mem_buf(encoded_string.c_str(), -1);
b64 = BIO_new(BIO_f_base64());
bio = BIO_push(b64, bio);
int length = BIO_read(bio, decoded_buffer, encoded_string.size());
BIO_free_all(bio);
return string(decoded_buffer, length);
}
int main()
{
// 初始化 GDI+ 库
GdiplusStartupInput gdiplusStartupInput;
ULONG_PTR gdiplusToken;
GdiplusStartup(&gdiplusToken, &gdiplusStartupInput, NULL);
// 从 base64 编码的字符串中获取图像数据
string base64_string = "iVBORw0KGgoAAAANSUhEUgAAAAUA";
string decoded_string = base64_decode(base64_string);
const char* data = decoded_string.c_str();
int data_size = decoded_string.size();
// 将二进制数据写入文件中
ofstream image_file("image.png", ios::out | ios::binary);
image_file.write(data, data_size);
image_file.close();
// 加载图像并在窗口中展示
Gdiplus::Image* image = new Gdiplus::Image(L"image.png");
int width = image->GetWidth();
int height = image->GetHeight();
HWND hwnd = CreateWindowExW(
WS_EX_CLIENTEDGE,
L"STATIC",
L"Image",
WS_VISIBLE | WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, width, height,
NULL, NULL, GetModuleHandle(NULL), NULL);
HDC hdc = GetDC(hwnd);
Graphics graphics(hdc);
graphics.DrawImage(image, 0, 0, width, height);
// 消息循环
MSG msg;
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
// 释放资源
delete image;
ReleaseDC(hwnd, hdc);
GdiplusShutdown(gdiplusToken);
return 0;
}
```
请注意,这段代码使用了OpenSSL库来进行base64解码,需要在编译时链接该库。在Windows系统上,我们还需要链接GDIPlus库和Crypt32库。您可以使用以下命令进行编译:
```
cl main.cpp /EHsc /DUNICODE /D_UNICODE gdiplus.lib crypt32.lib
```
在编译时需要指定 `/DUNICODE /D_UNICODE` 宏定义,以支持 Unicode 字符集。
阅读全文
相关推荐
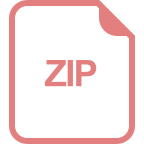
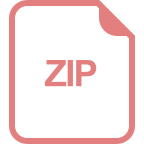
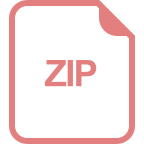
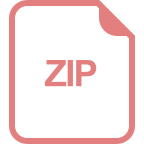
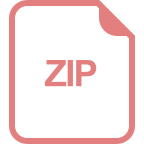
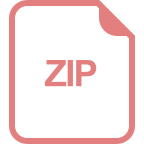
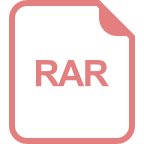
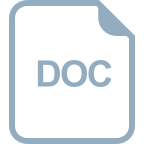
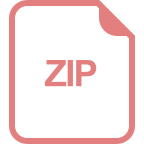
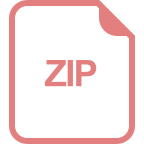
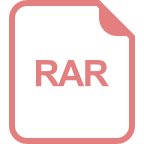
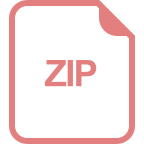
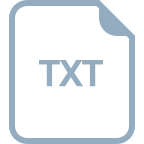
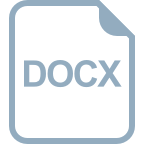
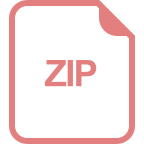
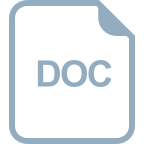
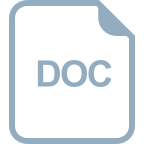