用C++写点云配准程序
时间: 2023-12-27 07:51:53 浏览: 28
以下是一个简单的点云配准程序,它使用ICP算法(最近点匹配算法)来将两个点云对齐。
首先,我们需要定义一个点的数据结构:
```
typedef struct Point {
double x;
double y;
double z;
} Point;
```
然后,我们定义一个函数来计算两个点云之间的欧氏距离:
```
double euclidean_distance(Point p1, Point p2) {
double dx = p1.x - p2.x;
double dy = p1.y - p2.y;
double dz = p1.z - p2.z;
return sqrt(dx*dx + dy*dy + dz*dz);
}
```
接下来,我们定义一个函数来计算一个点在另一个点云中的最近邻点:
```
Point find_nearest_neighbor(Point p, Point* cloud, int cloud_size) {
Point nearest_neighbor;
double min_distance = INFINITY;
for (int i = 0; i < cloud_size; i++) {
double distance = euclidean_distance(p, cloud[i]);
if (distance < min_distance) {
min_distance = distance;
nearest_neighbor = cloud[i];
}
}
return nearest_neighbor;
}
```
然后,我们定义一个函数来计算两个点云之间的误差平方和(即,对于每个点,计算该点在另一个点云中的最近邻点,然后计算这两个点之间的距离的平方和):
```
double calculate_error(Point* source_cloud, Point* target_cloud, int cloud_size) {
double error = 0.0;
for (int i = 0; i < cloud_size; i++) {
Point nearest_neighbor = find_nearest_neighbor(source_cloud[i], target_cloud, cloud_size);
double distance = euclidean_distance(source_cloud[i], nearest_neighbor);
error += distance*distance;
}
return error;
}
```
最后,我们定义一个函数来执行ICP算法。这个函数接受两个点云和它们的大小,然后返回对齐后的源点云。
```
Point* icp(Point* source_cloud, Point* target_cloud, int cloud_size) {
Point* aligned_cloud = malloc(cloud_size * sizeof(Point));
memcpy(aligned_cloud, source_cloud, cloud_size * sizeof(Point));
double error_threshold = 0.001;
double error = INFINITY;
int iterations = 0;
int max_iterations = 100;
while (error > error_threshold && iterations < max_iterations) {
// 计算每个点在目标点云中的最近邻点
for (int i = 0; i < cloud_size; i++) {
Point nearest_neighbor = find_nearest_neighbor(aligned_cloud[i], target_cloud, cloud_size);
aligned_cloud[i] = nearest_neighbor;
}
// 计算误差
double new_error = calculate_error(aligned_cloud, target_cloud, cloud_size);
printf("Iteration %d: error = %f\n", iterations, new_error);
// 检查误差是否有所减少
if (new_error < error) {
error = new_error;
} else {
break;
}
iterations++;
}
return aligned_cloud;
}
```
现在我们可以调用icp函数来执行点云配准:
```
int main() {
Point source_cloud[] = {{1.0, 2.0, 3.0}, {4.0, 5.0, 6.0}, {7.0, 8.0, 9.0}};
Point target_cloud[] = {{2.0, 3.0, 4.0}, {5.0, 6.0, 7.0}, {8.0, 9.0, 10.0}};
int cloud_size = 3;
Point* aligned_cloud = icp(source_cloud, target_cloud, cloud_size);
// 在这里,我们可以将对齐后的点云输出到文件或显示在屏幕上
// ...
free(aligned_cloud);
return 0;
}
```
当然,这只是一个简单的例子,实际的点云配准程序可能需要更多的功能和优化。
相关推荐
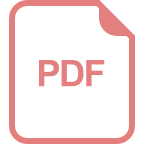














