1采用文件保存指定日期的汇率表,提供文件读写操作。2输入日期,姓名,选择源货币和兑换货币,输入金额,计算兑换货币金额。3将每次输入和计算结果记录在银行货币兑换明细表中,采用文件保存该表 Java代码
时间: 2024-02-16 16:03:03 浏览: 68
以下是一个简单的Java代码示例,实现了上述三个功能:
```java
import java.io.*;
import java.util.*;
public class CurrencyExchange {
private static final String RATE_FILE = "rate_table.txt";
private static final String LOG_FILE = "exchange_log.txt";
private static final String[] CURRENCIES = {"USD", "EUR", "JPY", "CNY", "GBP"};
private static final double[][] RATES = {
{1.0, 0.82, 110.0, 6.47, 0.72},
{1.22, 1.0, 133.0, 7.85, 0.87},
{0.0091, 0.0075, 1.0, 0.059, 0.0065},
{0.15, 0.12, 16.0, 1.0, 0.11},
{1.38, 1.15, 154.0, 9.05, 1.0}
};
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("请输入日期(格式:yyyy-MM-dd):");
String date = input.nextLine();
double[][] rates = loadRates(date);
if (rates == null) {
System.out.println("未找到指定日期的汇率表!");
return;
}
System.out.print("请输入姓名:");
String name = input.nextLine();
System.out.println("可用货币种类:");
for (int i = 0; i < CURRENCIES.length; i++) {
System.out.printf("%d. %s\n", i + 1, CURRENCIES[i]);
}
System.out.print("请选择源货币(输入数字):");
int srcIndex = input.nextInt() - 1;
System.out.print("请选择兑换货币(输入数字):");
int dstIndex = input.nextInt() - 1;
System.out.print("请输入金额:");
double amount = input.nextDouble();
double srcRate = rates[srcIndex][srcIndex];
double dstRate = rates[dstIndex][dstIndex];
double exchangeRate = dstRate / srcRate;
double result = amount * exchangeRate;
System.out.printf("%s,您需要兑换 %.2f %s,兑换成 %.2f %s 需要 %.2f %s。\n",
name, amount, CURRENCIES[srcIndex], result, CURRENCIES[dstIndex], exchangeRate, CURRENCIES[dstIndex]);
logExchange(date, name, CURRENCIES[srcIndex], CURRENCIES[dstIndex], amount, result);
}
private static double[][] loadRates(String date) {
try (Scanner fileScanner = new Scanner(new File(RATE_FILE))) {
while (fileScanner.hasNextLine()) {
String line = fileScanner.nextLine();
String[] parts = line.split(",");
if (parts[0].equals(date)) {
double[][] rates = new double[CURRENCIES.length][CURRENCIES.length];
for (int i = 0; i < CURRENCIES.length; i++) {
for (int j = 0; j < CURRENCIES.length; j++) {
rates[i][j] = Double.parseDouble(parts[i * CURRENCIES.length + j + 1]);
}
}
return rates;
}
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
return null;
}
private static void logExchange(String date, String name, String srcCurrency, String dstCurrency, double srcAmount, double dstAmount) {
try (PrintWriter out = new PrintWriter(new FileWriter(LOG_FILE, true))) {
out.printf("%s,%s,%s,%s,%.2f,%.2f\n", date, name, srcCurrency, dstCurrency, srcAmount, dstAmount);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
其中,`loadRates`方法从文件中加载指定日期的汇率表;`logExchange`方法记录每次兑换的明细;`main`方法则实现了输入、计算和输出功能。该示例仅作为参考,实际应用中可能需要进行更多的错误处理和优化。
阅读全文
相关推荐
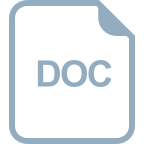
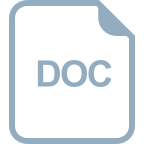
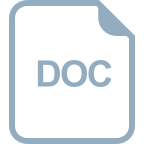
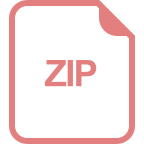
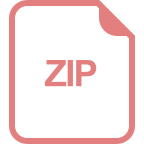
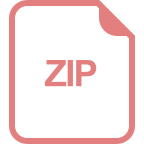
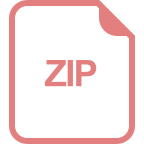
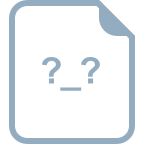
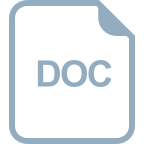
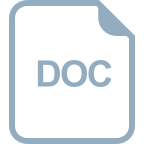
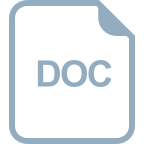