用Qt编译有界面的无重复字符的最长子串
时间: 2024-05-11 13:18:32 浏览: 14
以下是一个基于Qt的无重复字符的最长子串的实现,包含了一个简单的界面。
首先,我们需要一个函数来计算给定字符串的最长无重复字符的子串:
```c++
QString longestSubstringWithoutRepeatingCharacters(const QString& str)
{
QString longestSubstring;
QString currentSubstring;
QSet<QChar> uniqueCharacters;
for (const QChar& c : str) {
if (uniqueCharacters.contains(c)) {
if (currentSubstring.length() > longestSubstring.length()) {
longestSubstring = currentSubstring;
}
while (!currentSubstring.isEmpty() && currentSubstring.at(0) != c) {
uniqueCharacters.remove(currentSubstring.at(0));
currentSubstring.remove(0, 1);
}
currentSubstring.remove(0, 1);
}
uniqueCharacters.insert(c);
currentSubstring.append(c);
}
if (currentSubstring.length() > longestSubstring.length()) {
longestSubstring = currentSubstring;
}
return longestSubstring;
}
```
接下来,我们需要一个Qt界面来获取输入和显示输出。我们可以使用Qt的QWidget和QLineEdit类来实现这一点:
```c++
class MainWindow : public QWidget
{
Q_OBJECT
public:
MainWindow(QWidget* parent = nullptr);
~MainWindow();
private slots:
void onTextChanged(const QString& text);
private:
QLineEdit* m_inputLineEdit;
QLineEdit* m_outputLineEdit;
};
```
```c++
MainWindow::MainWindow(QWidget* parent)
: QWidget(parent)
{
m_inputLineEdit = new QLineEdit(this);
m_outputLineEdit = new QLineEdit(this);
QVBoxLayout* layout = new QVBoxLayout(this);
layout->addWidget(m_inputLineEdit);
layout->addWidget(m_outputLineEdit);
connect(m_inputLineEdit, &QLineEdit::textChanged, this, &MainWindow::onTextChanged);
}
MainWindow::~MainWindow()
{
}
void MainWindow::onTextChanged(const QString& text)
{
QString longestSubstring = longestSubstringWithoutRepeatingCharacters(text);
m_outputLineEdit->setText(longestSubstring);
}
```
最后,我们可以使用Qt的qmake工具来生成Makefile并编译我们的应用程序。在项目目录下创建一个名为“longestsubstring.pro”的文件,内容如下:
```
QT += widgets
TARGET = longestsubstring
SOURCES += main.cpp
```
然后在终端中运行以下命令:
```
qmake longestsubstring.pro
make
```
这将生成一个可执行文件“longestsubstring”以及所有必需的库文件。现在,我们可以运行我们的应用程序并测试它。
相关推荐
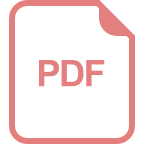
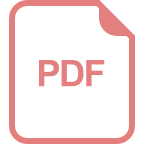
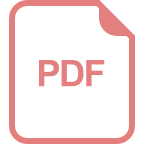
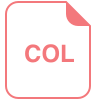

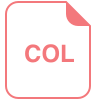
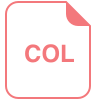
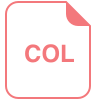









