c# 自定义控件 根据圆 的角度求控件的高度 设置自定义控件的长和宽 例子
时间: 2024-09-12 22:12:17 浏览: 21
在C#中创建自定义控件时,可以通过重写控件的`OnPaint`方法来自定义控件的绘制逻辑。如果需要根据圆的角度来计算控件的高度,可以使用三角函数来实现。下面是一个简单的例子,展示了如何创建一个自定义控件,并根据圆的直径来设置控件的长和宽,同时根据圆的角度来调整控件的高度。
```csharp
using System;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Windows.Forms;
public class CustomCircleControl : Control
{
private float _angle;
// 属性允许外部设置角度
public float Angle
{
get { return _angle; }
set
{
_angle = value;
// 角度变化时,重绘控件
Invalidate();
}
}
public CustomCircleControl()
{
// 设置控件的默认尺寸
Size = new Size(200, 200);
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
Graphics g = e.Graphics;
// 计算控件的中心点
int centerX = Width / 2;
int centerY = Height / 2;
// 计算圆的半径
int radius = Math.Min(centerX, centerY);
// 计算圆的直径,即控件的宽度和高度(这里保持长宽一致)
int diameter = radius * 2;
// 创建圆的路径
using (GraphicsPath path = new GraphicsPath())
{
path.AddEllipse(0, 0, diameter, diameter);
// 将路径用于区域,以便裁剪绘图区域
Region = new Region(path);
}
// 设置画刷颜色
using (Brush brush = new SolidBrush(Color.FromArgb(255, 255, 192, 0)))
{
// 根据角度计算高度,假设角度从水平线开始逆时针增加
int calculatedHeight = (int)(diameter * Math.Cos(_angle * Math.PI / 180));
// 计算高度的偏移量
int heightOffset = (diameter - calculatedHeight) / 2;
// 画一个矩形以显示高度变化的效果
g.FillRectangle(brush, 0, heightOffset, diameter, calculatedHeight);
}
}
}
// 使用示例
public class Program
{
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
// 创建自定义控件实例并设置窗体
CustomCircleControl control = new CustomCircleControl();
control.Angle = 30; // 设置角度
Form form = new Form { Controls = { control } };
Application.Run(form);
}
}
```
在这个例子中,`CustomCircleControl`类继承自`Control`类,并重写了`OnPaint`方法来绘制圆和根据角度计算高度的矩形。`Angle`属性允许外部设置角度,从而触发控件的重绘。
相关推荐
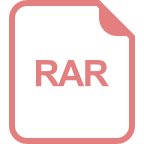
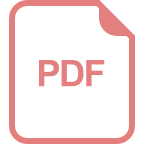














