如何在其他编程语言中实现字符串比较和差异展示?
时间: 2024-09-10 18:27:52 浏览: 94
在不同的编程语言中实现字符串比较和差异展示的方法各有特点,但总体思路是通过比较两个字符串的内容来找出它们之间的不同。下面我将分别介绍在几种常见编程语言中实现这一功能的方法。
1. Python:Python中可以使用 difflib 库来比较字符串,并找出差异。difflib 提供了 SequenceMatcher 类用于比较两个序列的相似度,并可以通过它找到两个字符串之间的差异。
示例代码:
```python
import difflib
str1 = "Hello world"
str2 = "Hella world"
seq_matcher = difflib.SequenceMatcher(None, str1, str2)
diff = seq_matcher.get_opcodes()
for tag, i1, i2, j1, j2 in diff:
if tag == 'replace':
print(f"在 {i1}:{i2} 删除了,{j1}:{j2} 插入了")
elif tag == 'delete':
print(f"在 {i1}:{i2} 删除了")
elif tag == 'insert':
print(f"在 {j1}:{j2} 插入了")
elif tag == 'equal':
print(f"在 {i1}:{i2} 和 {j1}:{j2} 相同")
elif tag == 'noop':
print(f"在 {i1}:{i2} 未改变")
```
2. JavaScript:在JavaScript中,可以通过逐字符比较来实现字符串差异的展示,但更为常见的是使用第三方库,如diff-match-patch,它提供了强大的字符串比较和差异展示功能。
示例代码:
```javascript
const diff_match_patch = require('diff-match-patch');
const dmp = new diff_match_patch();
let str1 = "Hello world";
let str2 = "Hella world";
let diffs = dmp.diff_main(str1, str2);
dmp.diff_cleanupSemantic(diffs);
diffs.forEach(diff => {
if (diff[0] === 1) {
console.log(`+ ${diff[1]}`);
} else if (diff[0] === -1) {
console.log(`- ${diff[1]}`);
} else {
console.log(` ${diff[1]}`);
}
});
```
3. Java:Java中没有内置的字符串比较库,但是可以手动实现一个简单的算法来比较两个字符串并找出差异,或者使用第三方库如Apache Commons Lang的StringUtils类中的方法。
示例代码:
```java
import org.apache.commons.lang3.StringUtils;
String str1 = "Hello world";
String str2 = "Hella world";
String diff = StringUtils.difference(str1, str2);
System.out.println("差异内容: " + diff);
```
阅读全文
相关推荐




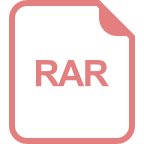

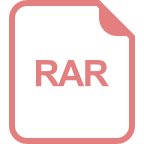











