Linux c++实现多个照片贴成人头
时间: 2023-10-27 14:03:58 浏览: 41
实现多个照片贴成一个人头可以使用图像拼接技术。以下是一个基本的实现步骤:
1. 加载多个照片成为图像对象
2. 提取每个照片中的人脸并对齐
3. 将所有人脸放入同一张图像中
4. 进行图像拼接并调整拼接后的图像
这个过程需要使用到图像处理库和人脸识别库。常见的图像处理库包括OpenCV和ImageMagick,人脸识别库包括OpenCV、Dlib和FaceNet等。
以下是一个使用OpenCV和Dlib实现人脸拼接的示例代码:
```c
#include <stdio.h>
#include <opencv2/opencv.hpp>
#include <dlib/opencv.h>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing.h>
using namespace cv;
using namespace dlib;
int main(int argc, char** argv)
{
// Load images
Mat image1 = imread("image1.jpg");
Mat image2 = imread("image2.jpg");
Mat image3 = imread("image3.jpg");
Mat image4 = imread("image4.jpg");
// Create a vector of images
std::vector<Mat> images = { image1, image2, image3, image4 };
// Load face detection model
frontal_face_detector detector = get_frontal_face_detector();
shape_predictor predictor;
deserialize("shape_predictor_68_face_landmarks.dat") >> predictor;
// Extract faces and align
std::vector<Mat> faces;
for (auto img : images) {
// Convert to dlib format
cv_image<bgr_pixel> cimg(img);
// Detect faces
std::vector<rectangle> dets = detector(cimg);
// Extract and align faces
for (auto det : dets) {
full_object_detection shape = predictor(cimg, det);
Mat face = dlib::toMat(cimg(shape.get_rect()));
faces.push_back(face);
}
}
// Create a new image and paste faces
Mat new_image(600, 600, CV_8UC3, Scalar(255, 255, 255));
int x = 0;
for (auto face : faces) {
resize(face, face, Size(200, 200));
Rect roi(x, 200, 200, 200);
face.copyTo(new_image(roi));
x += 200;
}
// Resize new image
resize(new_image, new_image, Size(400, 400));
// Save result
imwrite("result.jpg", new_image);
return 0;
}
```
在这个示例中,我们加载了四个照片,并使用Dlib库中的人脸检测器和特征点检测器提取出每个照片中的人脸并进行对齐。然后,我们创建一个新的图像并将每个人脸贴到新图像中。最后,我们对新图像进行调整并保存结果。
相关推荐
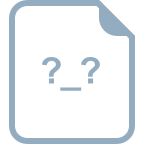
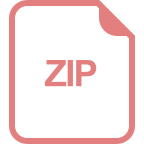














