<script setup>用这个方法写一遍
时间: 2024-02-09 17:12:17 浏览: 66
好的,下面是使用 `<script setup>` 写法的示例代码,来实现父组件中的数组传递,并在子组件中进行修改:
父组件:
```html
<template>
<div>
<input v-for="(item, index) in list" :key="index" v-model="list[index]" />
<ChildComponent :list="list" @update:list="list = $event" />
</div>
</template>
<script setup>
import { ref } from "vue";
import ChildComponent from "./ChildComponent.vue";
const list = ref(["apple", "banana", "orange"]);
export default {
components: {
ChildComponent,
},
};
</script>
```
在上面的代码中,我们使用了 `<script setup>` 语法糖来定义了一个响应式变量 `list`,并将其作为 prop 传递给了子组件 `ChildComponent`。
子组件:
```html
<template>
<div>
<input v-for="(item, index) in list" :key="index" v-model="list[index]" @input="updateList" />
</div>
</template>
<script setup>
import { ref, watch, emit } from "vue";
const props = defineProps({
list: {
type: Array,
required: true,
},
});
const list = ref(props.list);
const updateList = () => {
emit("update:list", list.value);
};
watch(props, () => {
list.value = props.list;
});
export default {
props,
list,
updateList,
};
</script>
```
在上面的代码中,我们首先使用 `defineProps` 函数来定义了一个 prop `list`,然后使用 `ref` 函数定义了一个响应式变量 `list` 来接收这个数组。
接着,我们定义了一个方法 `updateList`,用于在 input 中修改 `list` 的值,并将其通过 `emit` 函数传递回父组件。
最后,我们使用 `watch` 函数来监听父组件传递的 `list` 的变化,并更新子组件中的 `list` 值。
这样,我们就可以使用 `<script setup>` 写法来实现父组件中的数组传递,并在子组件中进行修改。需要注意的是,`<script setup>` 写法需要使用 `defineProps` 函数来定义 props,使用 `emit` 函数来触发事件,以及使用 `watch` 函数来监听 props 的变化。
阅读全文
相关推荐
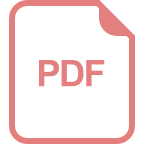
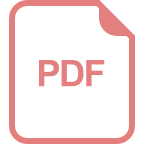
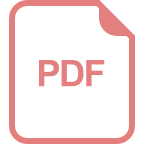
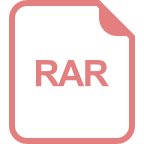
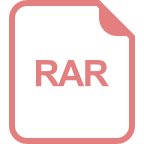
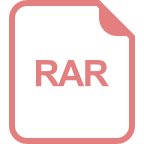
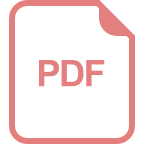
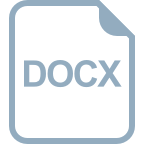
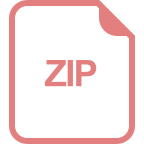
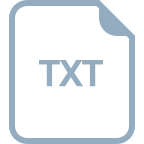
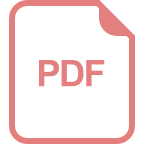
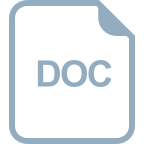
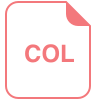
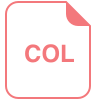
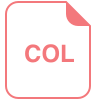
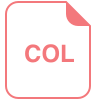
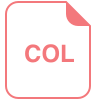
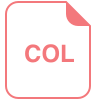