用c语言写图书管理系统
时间: 2023-05-14 17:05:04 浏览: 101
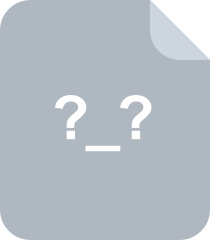
用C语言写的图书管理系统

好的,我可以为您提供一个简单的图书管理系统的C语言代码示例:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100
struct Book {
char title[50];
char author[50];
int id;
};
struct Library {
struct Book books[MAX_BOOKS];
int num_books;
};
void add_book(struct Library *lib, char *title, char *author, int id) {
if (lib->num_books >= MAX_BOOKS) {
printf("Error: library is full\n");
return;
}
struct Book new_book;
strcpy(new_book.title, title);
strcpy(new_book.author, author);
new_book.id = id;
lib->books[lib->num_books] = new_book;
lib->num_books++;
printf("Book added successfully\n");
}
void remove_book(struct Library *lib, int id) {
int i;
for (i = 0; i < lib->num_books; i++) {
if (lib->books[i].id == id) {
int j;
for (j = i; j < lib->num_books - 1; j++) {
lib->books[j] = lib->books[j+1];
}
lib->num_books--;
printf("Book removed successfully\n");
return;
}
}
printf("Error: book not found\n");
}
void print_books(struct Library *lib) {
int i;
for (i = 0; i < lib->num_books; i++) {
printf("Title: %s\n", lib->books[i].title);
printf("Author: %s\n", lib->books[i].author);
printf("ID: %d\n", lib->books[i].id);
printf("\n");
}
}
int main() {
struct Library lib;
lib.num_books = 0;
int choice;
do {
printf("1. Add book\n");
printf("2. Remove book\n");
printf("3. Print books\n");
printf("4. Quit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
{
char title[50];
char author[50];
int id;
printf("Enter title: ");
scanf("%s", title);
printf("Enter author: ");
scanf("%s", author);
printf("Enter ID: ");
scanf("%d", &id);
add_book(&lib, title, author, id);
break;
}
case 2:
{
int id;
printf("Enter ID: ");
scanf("%d", &id);
remove_book(&lib, id);
break;
}
case 3:
{
print_books(&lib);
break;
}
case 4:
{
printf("Goodbye!\n");
break;
}
default:
{
printf("Invalid choice\n");
break;
}
}
} while (choice != 4);
return 0;
}
阅读全文
相关推荐



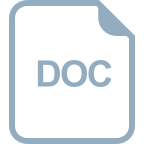
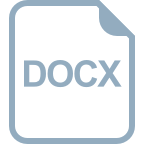
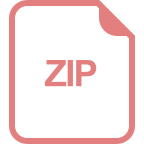
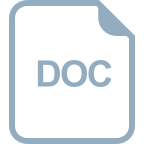
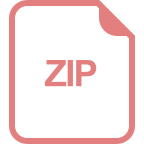
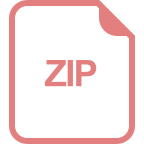
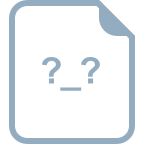


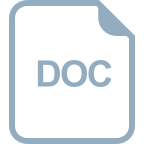