elasticsearchrepository使用
时间: 2023-05-31 19:20:28 浏览: 139
### 回答1:
ElasticsearchRepository是Spring Data Elasticsearch提供的一个接口,用于简化与Elasticsearch的交互。它提供了一组CRUD操作,以及一些常见的查询方法,使得我们可以更加方便地操作Elasticsearch。
使用ElasticsearchRepository,我们需要定义一个继承自它的接口,并指定实体类和主键类型。然后,我们就可以在接口中定义我们需要的方法,比如根据某个字段查询数据、分页查询等等。
在使用ElasticsearchRepository之前,我们需要先在Spring Boot中配置Elasticsearch的连接信息。具体的配置方式可以参考Spring Data Elasticsearch官方文档。
总之,ElasticsearchRepository是一个非常方便的工具,可以帮助我们更加轻松地操作Elasticsearch。
### 回答2:
Elasticsearch是一款非常流行的开源搜索引擎,它在搜索和分析领域都有着广泛的应用。而ElasticsearchRepository是Spring Data Elasticsearch框架提供的一个封装了Elasticsearch的仓库类,它将Elasticsearch的操作转换成Java方法,方便程序员进行操作。
使用ElasticsearchRepository需要以下几个步骤:
1.在pom.xml文件中加入spring-data-elasticsearch的依赖
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-elasticsearch</artifactId>
<version>4.3.3</version>
</dependency>
2.创建一个ElasticsearchRepository接口
public interface BookRepository extends ElasticsearchRepository<Book,String>{
}
其中Book是索引类型(index type),String是主键类型。
3.在BookRepository中定义自己的查询方法
public interface BookRepository extends ElasticsearchRepository<Book,String>{
List<Book> findByName(String name);
Page<Book> findByAuthor(String author, Pageable pageable);
}
在定义零散方法时需要按照Spring Data的规范来编写,例如:findXXXByXXX等方式,Spring会自动将方法名解析成ES查询语句,而且参数也可以用Spring的Query注解来指定查询条件。
4.将BookRepository注入到业务中
@Autowired
private BookRepository bookRepository;
5.使用BookRepository中的方法进行ES的操作
List<Book> books = bookRepository.findByName("Thinking in Java");
Page<Book> page = bookRepository.findByAuthor("Bruce Eckel", PageRequest.of(0,10));
通过以上介绍,可以看出使用ElasticsearchRepository可以更加方便地进行Elasticsearch操作。当然,如何查询和使用Index,Type等操作,并不在本文的讨论范围之内。还是需要多了解Elasticsearch本身的操作以及Spring Data Elasticsearch框架的使用。
### 回答3:
ElasticsearchRepository是Spring Data Elasticsearch提供的一个库,用于简化与Elasticsearch交互的操作。它提供了一个抽象的接口,通过继承它,我们可以轻松地对Elasticsearch进行CRUD操作。
首先,我们需要在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-elasticsearch</artifactId>
<version>4.1.0</version>
</dependency>
```
然后,我们需要定义一个实体类,使用@Document注解将其映射到Elasticsearch索引中:
```
@Document(indexName = "my-index", type = "my-type")
public class Person {
@Id
private String id;
private String name;
private int age;
// getters and setters
}
```
在这个例子里,我们将这个实体类映射到了名为“my-index”、类型为“my-type”的索引中。
接下来,我们需要定义一个继承自ElasticsearchRepository的接口:
```
public interface PersonRepository extends ElasticsearchRepository<Person, String> {
}
```
在这个例子里,我们定义了一个PersonRepository接口,它继承自ElasticsearchRepository。注意,我们需要传入两个类型参数,第一个参数为实体类的类型,第二个参数为实体类主键的类型。
现在,我们就可以在我们的应用中使用PersonRepository了。它已经继承了ElasticsearchRepository中的CRUD方法,我们可以使用它们来进行简单的CRUD操作:
```
@Autowired
private PersonRepository personRepository;
// create
Person person = new Person();
person.setName("John");
person.setAge(30);
personRepository.save(person);
// read
Optional<Person> optionalPerson = personRepository.findById("1");
Person person = optionalPerson.isPresent() ? optionalPerson.get() : null;
// update
person.setName("Johnny");
personRepository.save(person);
// delete
personRepository.deleteById("1");
```
总的来说,使用ElasticsearchRepository可以大大简化与Elasticsearch的交互操作,提高开发效率。
阅读全文
相关推荐
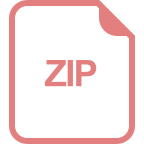
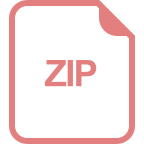















