DS18B20温度传感器的数据为什么乘0.625
时间: 2024-05-30 15:11:52 浏览: 74
DS18B20温度传感器的原始数据是一个16位的二进制数,其中前15位表示温度数据,最后一位表示小数点。这个16位二进制数需要转换为实际的温度值。
DS18B20温度传感器的精度是0.0625°C,也就是说,每个LSB(最小可测量单位)代表0.0625°C。因此,将传感器读取的16位二进制数乘以0.0625,就可以得到实际的温度值,单位为°C。
为了方便计算,可以将0.0625写成0.625/10,这样就可以将16位二进制数乘以0.625,然后除以10,得到实际的温度值。
相关问题
ds18b20温度传感器代码51
以下是基于51单片机的DS18B20温度传感器代码:
```c
#include<reg51.h>
#include<intrins.h>
#define uchar unsigned char
#define uint unsigned int
sbit DQ = P3^4; // DS18B20数据线
uchar code table[]={"0123456789"}; // 数码管显示字符
/* DS18B20初始化 */
void Init_DS18B20()
{
uchar i;
DQ = 1;
_nop_();
_nop_();
_nop_();
_nop_();
DQ = 0;
_nop_();
_nop_();
_nop_();
_nop_();
DQ = 1;
_nop_();
_nop_();
i = DQ;
_nop_();
_nop_();
}
/* DS18B20写一个字节 */
void Write_DS18B20(uchar dat)
{
uchar i;
for(i=0;i<8;i++)
{
DQ = 0;
_nop_();
_nop_();
DQ = dat&0x01;
_nop_();
_nop_();
_nop_();
_nop_();
DQ = 1;
dat = dat>>1;
}
}
/* DS18B20读一个字节 */
uchar Read_DS18B20()
{
uchar i,dat;
for(i=0;i<8;i++)
{
DQ = 0;
_nop_();
_nop_();
_nop_();
_nop_();
DQ = 1;
_nop_();
_nop_();
_nop_();
_nop_();
dat |= DQ<<i;
}
return dat;
}
/* DS18B20开始转换 */
void Start_DS18B20()
{
Init_DS18B20();
Write_DS18B20(0xcc); // 跳过ROM操作
Write_DS18B20(0x44); // 开始温度转换
}
/* DS18B20读温度 */
void Read_Temperature()
{
uchar i;
uint temp;
uchar a,b;
Init_DS18B20();
Write_DS18B20(0xcc); // 跳过ROM操作
Write_DS18B20(0xbe); // 读暂存器
a = Read_DS18B20(); // 读温度低8位
b = Read_DS18B20(); // 读温度高8位
temp = b;
temp <<= 8;
temp |= a;
temp = temp*0.625; // 转换为实际温度值,单位为摄氏度
for(i=0;i<4;i++)
{
P0 = 0xff; // 段选位全高
P2 = 0x7f; // 位选位选择第i+1位
P0 = table[temp%10]; // 个位数码管显示
temp /= 10;
Delay(5);
}
}
/* 延时函数 */
void Delay(uint i)
{
while(i--);
}
/* 主函数 */
void main()
{
while(1)
{
Start_DS18B20();
Delay(100);
Read_Temperature();
}
}
```
上述代码通过51单片机控制DS18B20温度传感器进行温度读取,并通过数码管进行显示。需要注意的是,DS18B20的数据线需要接在51单片机的P3.4口。
stm32ds18b20温度传感器代码
以下是使用STM32与DS18B20温度传感器通信的示例代码:
```c
#include "stm32f10x.h"
#include "ds18b20.h"
void delay_us(uint32_t nus)
{
uint32_t ticks = nus * (SystemCoreClock / 1000000);
while(ticks--);
}
void ds18b20_init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_12;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_SetBits(GPIOA, GPIO_Pin_12);
delay_us(2);
GPIO_ResetBits(GPIOA, GPIO_Pin_12);
delay_us(500);
GPIO_SetBits(GPIOA, GPIO_Pin_12);
delay_us(60);
}
void ds18b20_write_byte(uint8_t dat)
{
uint8_t i;
for(i=0; i<8; i++)
{
if(dat & 0x01)
{
GPIO_ResetBits(GPIOA, GPIO_Pin_12);
delay_us(10);
GPIO_SetBits(GPIOA, GPIO_Pin_12);
delay_us(60);
}
else
{
GPIO_ResetBits(GPIOA, GPIO_Pin_12);
delay_us(60);
GPIO_SetBits(GPIOA, GPIO_Pin_12);
delay_us(10);
}
dat >>= 1;
}
}
uint8_t ds18b20_read_byte(void)
{
uint8_t i, dat = 0;
for(i=0; i<8; i++)
{
GPIO_ResetBits(GPIOA, GPIO_Pin_12);
delay_us(2);
GPIO_SetBits(GPIOA, GPIO_Pin_12);
delay_us(8);
dat >>= 1;
if(GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_12))
dat |= 0x80;
delay_us(60);
}
return dat;
}
uint16_t ds18b20_read_temp(void)
{
uint8_t i;
uint8_t buf[9];
uint16_t temp;
ds18b20_init();
ds18b20_write_byte(0xCC);
ds18b20_write_byte(0x44);
delay_us(750);
ds18b20_init();
ds18b20_write_byte(0xCC);
ds18b20_write_byte(0xBE);
for(i=0; i<9; i++)
buf[i] = ds18b20_read_byte();
temp = buf[1];
temp <<= 8;
temp |= buf[0];
return temp;
}
int main(void)
{
uint16_t temp;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_13;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOC, &GPIO_InitStructure);
while(1)
{
temp = ds18b20_read_temp();
if(temp & 0x8000) //温度为负数
{
temp = ~temp + 1; //取反+1
GPIO_SetBits(GPIOC, GPIO_Pin_13);
}
else
GPIO_ResetBits(GPIOC, GPIO_Pin_13);
printf("Temperature: %d.%d C\n", temp>>4, (temp&0x0f)*625/100);
delay_us(2000000);
}
}
```
在本代码中,我们使用了PA12引脚与DS18B20进行通信,PC13引脚用于指示温度是否为负数。在主函数中,我们通过`ds18b20_read_temp()`函数读取温度值,并通过串口输出。需要注意的是,由于DS18B20的温度值带有小数部分,因此我们需要对读取到的数值进行转换,才能得到实际的温度值。
阅读全文
相关推荐
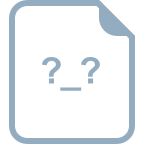
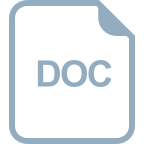
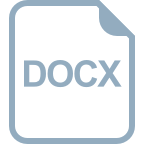










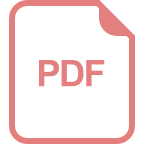

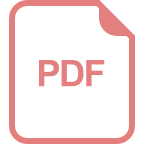
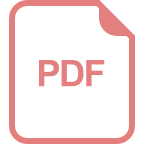