VB.NET 已经在窗体上建了picturebox1. 在picturebox1上绘制由多个点定义的几何图形, 包括多边形,基数样条,闭合基数样条的贝赛尔样条, 当在picturebox1中 单击鼠标左键时,将由序号和小圆圈点表示被点击位置, 当在picturebox1中单击右键时,则表示坐标点数组选择结束 如果要绘制贝赛尔曲线,则在选取4个点后单击鼠标右键来绘制。
时间: 2024-03-05 11:52:07 浏览: 63
以下是一个VB.NET的示例程序,实现了在PictureBox上绘制几何图形并响应鼠标事件。请注意,由于题目描述的比较简略,这个示例程序并没有实现所有的功能,但可以作为您开始编写代码的参考:
```
Public Class Form1
Dim g As Graphics
Dim points As New List(Of Point)
Dim bezierPoints As New List(Of Point)
Dim mode As Integer = 0 '0表示绘制多边形,1表示绘制基数样条,2表示绘制贝赛尔曲线
Dim bezierCount As Integer = 0 '表示贝赛尔曲线已经选择的点数
Private Sub PictureBox1_MouseDown(sender As Object, e As MouseEventArgs) Handles PictureBox1.MouseDown
If e.Button = MouseButtons.Left Then
'左键单击,添加点
points.Add(e.Location)
DrawPoints()
ElseIf e.Button = MouseButtons.Right Then
'右键单击,判断模式
If mode = 0 Then
'多边形模式,闭合图形
points.Add(points(0))
DrawPoints()
ElseIf mode = 2 AndAlso bezierCount < 4 Then
'贝赛尔曲线模式,选择点
bezierPoints.Add(e.Location)
bezierCount += 1
DrawPoints()
If bezierCount = 4 Then
'已选择4个点,绘制贝赛尔曲线
g.DrawBezier(Pens.Red, bezierPoints(0), bezierPoints(1), bezierPoints(2), bezierPoints(3))
bezierPoints.Clear()
bezierCount = 0
End If
End If
End If
End Sub
Private Sub DrawPoints()
'在PictureBox上绘制点
g.Clear(Color.White)
For i = 0 To points.Count - 1
g.FillEllipse(Brushes.Black, points(i).X - 3, points(i).Y - 3, 6, 6)
g.DrawString(i.ToString(), Me.Font, Brushes.Red, points(i).X, points(i).Y)
Next
If mode = 1 Then
'基数样条模式,绘制曲线
Dim curve = New System.Drawing.Drawing2D.GraphicsPath
curve.AddCurve(points.ToArray())
g.DrawPath(Pens.Blue, curve)
ElseIf mode = 2 AndAlso bezierCount > 0 Then
'贝赛尔曲线模式,绘制已选择的点
For i = 0 To bezierPoints.Count - 1
g.FillEllipse(Brushes.Red, bezierPoints(i).X - 2, bezierPoints(i).Y - 2, 4, 4)
Next
End If
PictureBox1.Image = g.Bitmap
End Sub
Private Sub RadioButton1_CheckedChanged(sender As Object, e As EventArgs) Handles RadioButton1.CheckedChanged
'选择多边形模式
mode = 0
points.Clear()
DrawPoints()
End Sub
Private Sub RadioButton2_CheckedChanged(sender As Object, e As EventArgs) Handles RadioButton2.CheckedChanged
'选择基数样条模式
mode = 1
points.Clear()
DrawPoints()
End Sub
Private Sub RadioButton3_CheckedChanged(sender As Object, e As EventArgs) Handles RadioButton3.CheckedChanged
'选择贝赛尔曲线模式
mode = 2
points.Clear()
bezierPoints.Clear()
bezierCount = 0
DrawPoints()
End Sub
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
g = PictureBox1.CreateGraphics()
End Sub
End Class
```
在这个示例程序中,我们在窗体上放置了三个RadioButton和一个PictureBox,RadioButton用于选择不同的绘制模式,PictureBox用于绘制图形。当用户在PictureBox上单击鼠标左键时,会添加一个点;当用户在PictureBox上单击鼠标右键时,会根据当前的模式来进行相应的操作。在绘制基数样条和贝赛尔曲线时,我们使用了.NET Framework提供的GraphicsPath类和DrawBezier方法来绘制曲线。
阅读全文
相关推荐






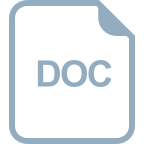







