在C#中现有一类型为List<List<T>>,T类型中有decimal类型属性,如何适用LINQ将其decimal类型属性精确小数点到2位,使用反射
时间: 2024-03-12 14:43:26 浏览: 27
你可以使用 LINQ 和反射来将 List<List<T>> 中的所有 decimal 类型属性保留 2 位小数:
```csharp
List<List<T>> listOfLists = ...;
listOfLists = listOfLists.Select(list =>
{
var newList = Activator.CreateInstance(list.GetType()) as IList;
foreach (var item in list)
{
var newItem = Activator.CreateInstance(item.GetType());
foreach (var prop in item.GetType().GetProperties())
{
if (prop.PropertyType == typeof(decimal))
{
var decimalValue = (decimal)prop.GetValue(item);
decimalValue = Math.Round(decimalValue, 2);
prop.SetValue(newItem, decimalValue);
}
else
{
prop.SetValue(newItem, prop.GetValue(item));
}
}
newList.Add(newItem);
}
return newList;
}).ToList();
```
上面的代码中,我们首先使用 Select 方法对 List<List<T>> 进行遍历,然后在内部遍历中,我们使用反射获取 T 类型的所有属性。如果属性的类型是 decimal,则使用 Math.Round 方法将其保留 2 位小数,并将新值设置回属性中;否则,将原始值设置回属性中。最后,我们将新的 List<T> 对象添加到一个新的 List<List<T>> 中,并将结果存储在一个新的 List<List<T>> 对象中。
需要注意的是,上面的代码使用了 Activator.CreateInstance 方法来创建新的 List<T> 和 T 对象,因此需要保证 T 类型和 List<T> 类型都具有无参数的公共构造函数。如果 T 类型和 List<T> 类型没有无参数的公共构造函数,则需要修改代码来适配具体的类型。
相关推荐
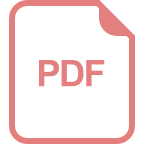
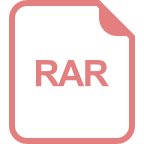
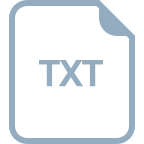










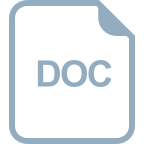
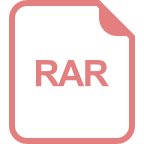
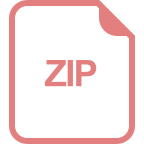
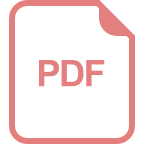
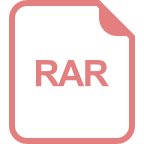
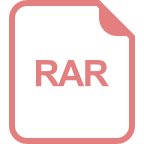