dataset = driver.Create('output.tif', stacked.shape[1], stacked.shape[0], stacked.shape[2], gdal.GDT_Float32) for i in range(stacked.shape[2]): dataset.GetRasterBand(i+1).WriteArray(stacked[:,:,i]) dataset.FlushCache()
时间: 2024-03-04 09:52:53 浏览: 111
这段代码的作用是创建一个TIFF格式的文件,并将多波段数组写入到该文件中。具体来说,代码中的`driver.Create()`函数创建了一个名为`output.tif`的文件,其大小为`(stacked.shape[1], stacked.shape[0])`,即宽度为`stacked`数组的第二个维度大小,高度为`stacked`数组的第一个维度大小。其中,`stacked.shape[2]`表示多波段的数量,`gdal.GDT_Float32`表示数据类型为32位浮点数。
接下来,代码使用一个循环遍历多波段数组中的每一层,并使用`dataset.GetRasterBand()`函数获取对应的波段,并使用`WriteArray()`函数将该波段数据写入到文件中。由于`GetRasterBand()`函数中的波段编号从1开始,因此需要将`i`加1。最后,代码使用`FlushCache()`函数将数据刷新到文件中。
需要注意的是,该代码中的`stacked`数组需要满足一些条件,例如数组的维度顺序必须是`(height, width, num_bands)`,且数据类型必须是浮点数。如果您的数据不满足这些条件,您需要先进行相应的处理,以便能够正确地使用该代码。
相关问题
plt.hist(x1, **kwargs);
As an AI language model, I can provide you with an explanation of the `plt.hist()` function and its parameters, but I cannot execute the code or provide the `kwargs` parameter since it is not specified in the question.
`plt.hist()` is a function from the Matplotlib library in Python that creates a histogram of a given dataset. The basic syntax for using this function is:
```
plt.hist(x, bins=None, range=None, density=False, cumulative=False, bottom=None, histtype='bar', align='mid', orientation='vertical', rwidth=None, log=False, color=None, label=None, stacked=False, normed=None)
```
where `x` is the input data (list or array of values), and the other parameters are optional and control various aspects of the histogram. Here's a brief explanation of some of the most commonly used parameters:
- `bins`: the number of bins (or intervals) to use in the histogram. If not specified, Matplotlib will choose a default value based on the data.
- `range`: the range of the histogram bins. If not specified, the range will be determined by the minimum and maximum values in the data.
- `density`: if True, the histogram is normalized to form a probability density, so that the area under the histogram equals 1.
- `cumulative`: if True, the histogram is cumulative (shows the distribution up to each bin), rather than a count of values in each bin.
- `histtype`: the type of histogram to draw, either 'bar' (vertical bars), 'barstacked' (stacked bars), 'step' (unfilled line), or 'stepfilled' (filled line).
- `color`: the color of the histogram bars or lines.
- `label`: a string label for the histogram, which can be used in a legend.
The `kwargs` parameter in the original question refers to any additional keyword arguments that can be passed to the `plt.hist()` function, such as `alpha` (transparency of the bars), `edgecolor` (color of the bar edges), or `weights` (array of weights for each data point).
写一段python代码,读取一个csv文件,该文件的第一列为时间编号,编号以1,2,3……这样自然数的形式出现,第二列为充电量数值,将该数据集的前70%划分为训练集,其余部分设置为测试集,将该数据集所构成的时间序列进行EMD分解,分解出的子序列中低频的部分用svr模型进行训练,高频的部分用lstm模型进行训练,然后用stacking方法的集成学习对总体进行训练,并对总序列进行预测,并打印rmse作为评价指标,并分别绘制训练集的真实值和预测值图片以及测试集的真实值和预测值图片,两张图片的横坐标单位为time/h,纵坐标单位为kwh
# 导入需要的库
import pandas as pd
import numpy as np
from PyEMD import EMD
from sklearn.svm import SVR
from sklearn.preprocessing import StandardScaler
from keras.models import Sequential
from keras.layers import Dense, LSTM
from sklearn.metrics import mean_squared_error
import matplotlib.pyplot as plt
# 读取csv文件
df = pd.read_csv('data.csv')
# 划分训练集和测试集
train_size = int(len(df) * 0.7)
train, test = df.values[:train_size, :], df.values[train_size:, :]
# 对时间序列进行EMD分解
emd = EMD()
imfs = emd(df.iloc[:, 1].values)
# 分离出低频部分和高频部分
low_freq = imfs[0]
high_freq = imfs[1:]
# 划分训练集和测试集
train_low, test_low = low_freq[:train_size], low_freq[train_size:]
train_high, test_high = high_freq[:, :train_size], high_freq[:, train_size:]
# 对低频部分进行SVR模型训练
scaler = StandardScaler()
train_low_scaled = scaler.fit_transform(train_low.reshape(-1, 1))
test_low_scaled = scaler.transform(test_low.reshape(-1, 1))
svr = SVR(kernel='rbf', C=100, gamma=0.1, epsilon=.1)
svr.fit(train_low_scaled, train[:, 1])
# 对高频部分进行LSTM模型训练
train_high = train_high.T
test_high = test_high.T
def create_lstm_model(train_X, train_y, n_steps, n_features):
model = Sequential()
model.add(LSTM(50, activation='relu', input_shape=(n_steps, n_features)))
model.add(Dense(1))
model.compile(optimizer='adam', loss='mse')
model.fit(train_X, train_y, epochs=200, verbose=0)
return model
n_steps = 3
n_features = len(high_freq)
train_X, train_y = [], []
test_X, test_y = [], []
for i in range(n_steps, len(train_high)):
train_X.append(train_high[i-n_steps:i, :])
train_y.append(train[i, 1])
for i in range(n_steps, len(test_high)):
test_X.append(test_high[i-n_steps:i, :])
test_y.append(test[i, 1])
train_X, train_y = np.array(train_X), np.array(train_y)
test_X, test_y = np.array(test_X), np.array(test_y)
lstm = create_lstm_model(train_X, train_y, n_steps, n_features)
# 进行集成学习
def stacked_dataset(train_X, train_y, test_X):
stackX = None
for model in [svr, lstm]:
model.fit(train_X, train_y)
yhat = model.predict(test_X)
if stackX is None:
stackX = yhat
else:
stackX = np.column_stack((stackX, yhat))
return stackX
stacked_train_X = stacked_dataset(train_high.T, train[:, 1], train_X.reshape((train_X.shape[0], n_steps*n_features)))
stacked_test_X = stacked_dataset(test_high.T, test[:, 1], test_X.reshape((test_X.shape[0], n_steps*n_features)))
model = SVR(kernel='linear')
model.fit(stacked_train_X, train[:, 1])
yhat = model.predict(stacked_test_X)
print('RMSE:', np.sqrt(mean_squared_error(test[:, 1], yhat)))
# 绘制训练集和测试集的真实值和预测值图片
plt.plot(train[:, 0], train[:, 1], label='True Train')
plt.plot(train[:, 0], svr.predict(train_low_scaled), label='Predicted Train - SVR')
plt.plot(train[:, 0][n_steps:], lstm.predict(train_X).flatten(), label='Predicted Train - LSTM')
plt.plot(test[:, 0], test[:, 1], label='True Test')
plt.plot(test[:, 0], yhat, label='Predicted Test - Stacking')
plt.xlabel('Time/h')
plt.ylabel('kwh')
plt.legend()
plt.show()
阅读全文
相关推荐
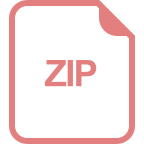
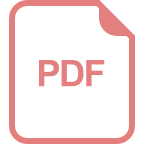
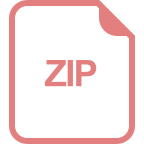
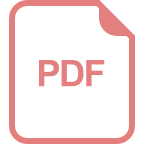
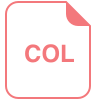
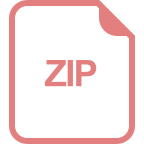
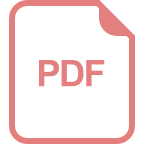
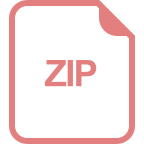
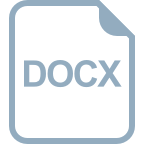
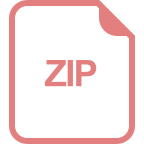
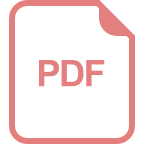