请用C语言写一个可以播放16khz、32khz、48khz音频采样频率的AAC播放器
时间: 2023-12-26 21:06:27 浏览: 52
这是一个比较复杂的问题,需要涉及到音频解码、音频处理、音频输出等多个方面。以下是一个大致的实现思路,供参考。
1. 首先需要使用一个AAC解码库,将AAC格式的音频数据解码成PCM数据。常用的AAC解码库有FFmpeg、OpenCORE等。
2. 解码后的PCM数据需要经过重采样,将其采样率转换为目标采样率。常用的重采样库有libsamplerate、soxr等。
3. 经过重采样后的PCM数据可以通过音频输出设备进行播放。可以使用系统提供的音频输出接口,如Windows的WASAPI、Linux的ALSA等。也可以使用第三方的音频输出库,如PortAudio、SDL等。
下面是一个简单的示例代码,演示如何使用FFmpeg解码AAC音频,并通过SDL输出。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <SDL.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswresample/swresample.h>
#define AUDIO_FRAME_SIZE 1024
int main(int argc, char *argv[]) {
if (argc < 2) {
fprintf(stderr, "Usage: %s <audio_file>\n", argv[0]);
return 1;
}
const char *audio_file = argv[1];
// 打开输入文件
AVFormatContext *fmt_ctx = NULL;
if (avformat_open_input(&fmt_ctx, audio_file, NULL, NULL) < 0) {
fprintf(stderr, "Error: could not open input file '%s'\n", audio_file);
return 1;
}
// 查找音频流
int audio_stream_index = av_find_best_stream(fmt_ctx, AVMEDIA_TYPE_AUDIO, -1, -1, NULL, 0);
if (audio_stream_index < 0) {
fprintf(stderr, "Error: could not find audio stream in input file '%s'\n", audio_file);
return 1;
}
// 获取音频解码器
AVCodecParameters *codec_params = fmt_ctx->streams[audio_stream_index]->codecpar;
AVCodec *codec = avcodec_find_decoder(codec_params->codec_id);
if (!codec) {
fprintf(stderr, "Error: unsupported audio codec '%s'\n", avcodec_get_name(codec_params->codec_id));
return 1;
}
// 打开音频解码器
AVCodecContext *codec_ctx = avcodec_alloc_context3(codec);
if (!codec_ctx) {
fprintf(stderr, "Error: could not allocate audio codec context\n");
return 1;
}
if (avcodec_parameters_to_context(codec_ctx, codec_params) < 0) {
fprintf(stderr, "Error: could not initialize audio codec context\n");
return 1;
}
if (avcodec_open2(codec_ctx, codec, NULL) < 0) {
fprintf(stderr, "Error: could not open audio codec\n");
return 1;
}
// 初始化重采样器
SwrContext *swr_ctx = swr_alloc_set_opts(NULL,
codec_ctx->channel_layout, AV_SAMPLE_FMT_S16, codec_ctx->sample_rate,
codec_ctx->channel_layout, codec_ctx->sample_fmt, codec_ctx->sample_rate,
0, NULL);
if (!swr_ctx) {
fprintf(stderr, "Error: could not allocate resampler context\n");
return 1;
}
if (swr_init(swr_ctx) < 0) {
fprintf(stderr, "Error: could not initialize resampler context\n");
return 1;
}
// 初始化SDL
if (SDL_Init(SDL_INIT_AUDIO) < 0) {
fprintf(stderr, "Error: could not initialize SDL\n");
return 1;
}
SDL_AudioSpec wanted_spec = {
.freq = 48000,
.format = AUDIO_S16SYS,
.channels = codec_ctx->channels,
.samples = AUDIO_FRAME_SIZE
};
SDL_AudioSpec spec;
SDL_AudioDeviceID dev = SDL_OpenAudioDevice(NULL, 0, &wanted_spec, &spec, 0);
if (!dev) {
fprintf(stderr, "Error: could not open SDL audio device\n");
return 1;
}
SDL_PauseAudioDevice(dev, 0);
// 解码并输出音频数据
AVPacket pkt;
av_init_packet(&pkt);
while (av_read_frame(fmt_ctx, &pkt) == 0) {
if (pkt.stream_index == audio_stream_index) {
AVFrame *frame = av_frame_alloc();
if (!frame) {
fprintf(stderr, "Error: could not allocate audio frame\n");
break;
}
int ret = avcodec_send_packet(codec_ctx, &pkt);
while (ret >= 0) {
ret = avcodec_receive_frame(codec_ctx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0) {
fprintf(stderr, "Error: could not decode audio frame\n");
break;
}
uint8_t *out_buf = malloc(frame->nb_samples * codec_ctx->channels * av_get_bytes_per_sample(AV_SAMPLE_FMT_S16));
if (!out_buf) {
fprintf(stderr, "Error: could not allocate output buffer\n");
break;
}
int out_samples = swr_convert(swr_ctx, &out_buf, frame->nb_samples,
(const uint8_t **)frame->extended_data, frame->nb_samples);
if (out_samples <= 0) {
free(out_buf);
continue;
}
SDL_QueueAudio(dev, out_buf, out_samples * codec_ctx->channels * av_get_bytes_per_sample(AV_SAMPLE_FMT_S16));
}
av_frame_free(&frame);
}
av_packet_unref(&pkt);
}
// 清理资源
swr_free(&swr_ctx);
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
SDL_CloseAudioDevice(dev);
SDL_Quit();
return 0;
}
```
该示例代码使用FFmpeg库进行AAC解码和PCM重采样,使用SDL库进行音频输出。可以通过编译链接FFmpeg和SDL库后运行。在Linux下可以使用以下命令编译:
```
gcc -o aacplayer aacplayer.c -lavcodec -lavformat -lswresample -lSDL2
```
在Windows下可以使用以下命令编译:
```
gcc -o aacplayer.exe aacplayer.c -lavcodec -lavformat -lswresample -lSDL2 -lws2_32
```
这里需要注意的是,不同操作系统下的编译链接方式可能有所不同,需要根据具体情况进行调整。
相关推荐
















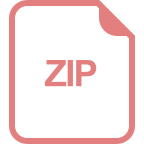
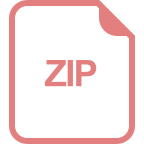
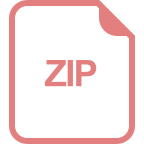