用python栈写一个迷宫问题 简单点
时间: 2024-05-29 14:04:23 浏览: 73
以下是一个简单的迷宫问题的Python栈实现:
```python
maze = [
[1, 1, 1, 1, 1, 1, 1],
[1, 0, 1, 0, 0, 0, 1],
[1, 0, 1, 0, 1, 0, 1],
[1, 0, 1, 0, 1, 1, 1],
[1, 0, 0, 0, 0, 0, 1],
[1, 0, 1, 1, 1, 0, 1],
[1, 1, 1, 1, 1, 1, 1]
]
# 定义迷宫地图
def print_maze(maze):
for row in maze:
print(row)
# 判断当前位置是否可以通过
def is_valid_pos(maze, x, y):
if x < 0 or x >= len(maze) or y < 0 or y >= len(maze[0]):
return False
if maze[x][y] == 1:
return False
return True
# 寻找迷宫的出口
def find_exit(maze, start_x, start_y, end_x, end_y):
stack = [(start_x, start_y)]
visited = set()
while stack:
x, y = stack.pop()
if (x, y) == (end_x, end_y):
return True
visited.add((x, y))
if is_valid_pos(maze, x-1, y) and (x-1, y) not in visited:
stack.append((x-1, y))
if is_valid_pos(maze, x+1, y) and (x+1, y) not in visited:
stack.append((x+1, y))
if is_valid_pos(maze, x, y-1) and (x, y-1) not in visited:
stack.append((x, y-1))
if is_valid_pos(maze, x, y+1) and (x, y+1) not in visited:
stack.append((x, y+1))
return False
# 测试
print_maze(maze)
print(find_exit(maze, 1, 1, 5, 5)) # True
print(find_exit(maze, 1, 1, 3, 3)) # False
```
在这个简单的实现中,我们使用了一个栈来存储当前位置和之前的位置,使用了一个集合来记录已经访问过的位置。在每次循环中,从栈中取出一个位置,并检查它是否是结束位置,如果是,则返回True,否则将其标记为已访问,并将其相邻的可通行位置添加到栈中。如果栈为空而未找到结束位置,则返回False。
阅读全文
相关推荐
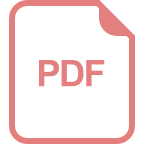
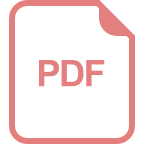
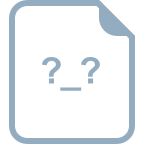















