用vue+setup写一个三级折叠面板加上下拉特效
时间: 2024-05-13 09:17:45 浏览: 8
好的,我可以给你提供一个简单的实现思路和代码示例。
首先,在 Vue 应用中,你需要使用 Vue 组件来实现三级折叠面板并添加下拉特效。接下来,我将展示如何使用 Vue 3 的 Composition API 和 setup() 函数来编写这个组件。
1. 创建组件
在你的 Vue 项目中,创建一个名为 "Accordion.vue" 的组件,并导入所需的 Vue 模块:
```
<template>
<div class="accordion">
<div class="accordion-item" v-for="(item, index) in items" :key="index">
<div class="accordion-header" @click="toggle(index)">
{{ item.title }}
<i :class="[isOpen(index) ? 'arrow-up' : 'arrow-down']"></i>
</div>
<div class="accordion-content" v-show="isOpen(index)">
<ul>
<li v-for="(subItem, subIndex) in item.items" :key="subIndex">
{{ subItem }}
</li>
</ul>
</div>
</div>
</div>
</template>
<script>
import { ref } from 'vue'
export default {
name: 'Accordion',
props: {
items: {
type: Array,
required: true
}
},
setup(props) {
const openItemIndex = ref(-1)
const isOpen = (index) => {
return openItemIndex.value === index
}
const toggle = (index) => {
if (isOpen(index)) {
openItemIndex.value = -1
} else {
openItemIndex.value = index
}
}
return {
openItemIndex,
isOpen,
toggle
}
}
}
</script>
<style>
/* 样式可以根据自己的需求进行调整 */
.accordion {
display: flex;
flex-direction: column;
}
.accordion-item {
margin-bottom: 10px;
}
.accordion-header {
display: flex;
justify-content: space-between;
align-items: center;
background-color: #eee;
padding: 10px;
cursor: pointer;
}
.accordion-header:hover {
background-color: #ddd;
}
.accordion-content {
padding: 10px;
background-color: #f8f8f8;
display: none;
}
.arrow-up {
border: solid black;
border-width: 0 3px 3px 0;
display: inline-block;
padding: 3px;
transform: rotate(-135deg);
-webkit-transform: rotate(-135deg);
}
.arrow-down {
border: solid black;
border-width: 0 3px 3px 0;
display: inline-block;
padding: 3px;
transform: rotate(45deg);
-webkit-transform: rotate(45deg);
}
ul {
margin: 0;
padding: 0;
list-style: none;
}
li {
margin-bottom: 5px;
}
</style>
```
2. 使用组件
现在你可以在你的 Vue 项目中使用 "Accordion" 组件了。在你的 Vue 单文件组件中,导入 "Accordion" 组件并将所需的数据传递给它:
```
<template>
<div>
<h1>三级折叠面板</h1>
<Accordion :items="items" />
</div>
</template>
<script>
import Accordion from './Accordion.vue'
export default {
components: {
Accordion
},
data() {
return {
items: [
{
title: '第一项',
items: ['1.1', '1.2', '1.3']
},
{
title: '第二项',
items: ['2.1', '2.2', '2.3']
},
{
title: '第三项',
items: ['3.1', '3.2', '3.3']
}
]
}
}
}
</script>
```
现在你可以在你的 Vue 应用中看到一个带有下拉特效的三级折叠面板。如果你点击面板标题,它将展开或收起它的子项。
相关推荐
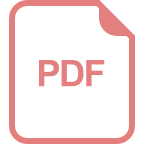
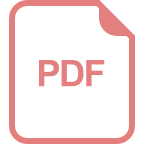
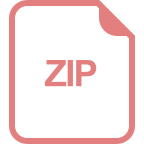






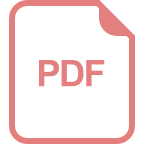
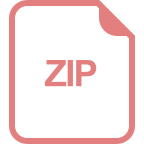
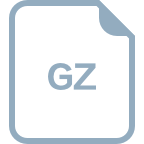
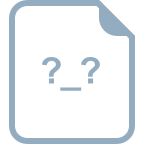