python ctypes 调BYTE *
时间: 2024-08-04 12:01:38 浏览: 118
Python的ctypes模块允许开发者直接与C库交互,包括处理C数据结构和函数。当你需要调用接受`BYTE*`(通常表示无类型的字节指针)的C函数时,你需要先创建一个ctypes数组(如`c_byte_array`)来代表这个内存区域。
例如,假设你在C中有一个接受`BYTE*`并执行某些操作的函数`my_c_function`:
```cpp
void my_c_function(BYTE* data, int length);
```
在Python中,你可以这样做:
```python
from ctypes import c_ubyte, create_string_buffer
# 创建一个包含指定长度字节的缓冲区
data = create_string_buffer(length)
# 将Python对象转换为ctypes字节指针
ptr_data = cast(data, POINTER(c_ubyte))
# 调用C函数,传递字节指针
my_c_function(ptr_data, length)
```
在这里,`c_ubyte`用于创建一个单字节的数组类型,`create_string_buffer`创建了一个指定长度的内存区域,`cast`则将Python内存区域转化为C可识别的指针形式。
相关问题
python ctypes数据类型
Python中的ctypes模块提供了一种与C语言兼容的数据类型,它允许Python代码调用动态链接库中的C函数。ctypes数据类型包括基本数据类型(如整数、浮点数、指针等)和复合数据类型(如结构体、联合体等)。以下是一些常见的ctypes数据类型及其对应的C语言数据类型:
- c_char:char
- c_wchar:wchar_t
- c_byte:signed char
- c_ubyte:unsigned char
- c_short:short
- c_ushort:unsigned short
- c_int:int
- c_uint:unsigned int
- c_long:long
- c_ulong:unsigned long
- c_longlong:long long
- c_ulonglong:unsigned long long
- c_float:float
- c_double:double
- c_void_p:void *
下面是一个例子,演示如何使用ctypes定义一个C语言中的结构体,并在Python中使用它:
```python
import ctypes
# 定义一个C语言中的结构体
class POINT(ctypes.Structure):
_fields_ = [("x", ctypes.c_int),
("y", ctypes.c_int)]
# 在Python中使用结构体
pt = POINT(10, 20)
print("x = {}, y = {}".format(pt.x, pt.y)) # 输出:x = 10, y = 20
```
python ctypes指针写入文件
可以使用Python的ctypes模块,将指针内容写入文件。具体步骤如下:
1. 打开文件,获取文件句柄。
```python
f = open('file.bin', 'wb')
```
2. 定义ctypes指针类型,并将其赋值为要写入文件的指针。
```python
from ctypes import *
# 定义指针类型
LPBYTE = POINTER(c_byte)
# 将要写入文件的指针赋值给指针类型变量
ptr = LPBYTE(0x12345678)
```
3. 使用ctypes内置的memmove函数,将指针内容写入文件。
```python
from ctypes import *
# 定义指针类型
LPBYTE = POINTER(c_byte)
# 将要写入文件的指针赋值给指针类型变量
ptr = LPBYTE(0x12345678)
# 将指针内容写入文件
memmove(f, ptr, sizeof(c_byte))
```
4. 关闭文件。
```python
f.close()
```
这样就可以将指针内容写入文件了。需要注意的是,写入文件的指针内容必须是有效的,否则可能会导致程序崩溃或写入无效数据。
阅读全文
相关推荐






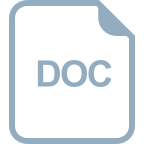









