子线程想访问父线程中的变量,java代码怎么写
时间: 2024-04-29 21:26:07 浏览: 124
可以使用Java中的ThreadLocal类来实现子线程访问父线程中的变量。具体代码如下:
在父线程中设置ThreadLocal变量:
```
public class ParentThread {
private static ThreadLocal<String> threadLocalVar = new ThreadLocal<>();
public static void main(String[] args) {
threadLocalVar.set("Hello World!");
...
}
}
```
在子线程中获取ThreadLocal变量:
```
public class ChildThread extends Thread {
public void run() {
String val = ParentThread.threadLocalVar.get();
...
}
}
```
在子线程中获取到的变量值就是父线程中设置的值。每个线程都有自己的ThreadLocal变量副本,修改不会影响其他线程的变量值,保证了线程安全性。
相关问题
子线程如何通过中断标志来通知父线程应该中断?给出java代码
子线程可以通过中断标志来通知父线程应该中断。具体的实现方式可以通过共享一个中断标志变量,然后在子线程中设置中断标志,父线程在执行过程中检查该标志并决定是否中断自己的执行。
下面是一个示例代码:
```
public class InterruptExample {
private static volatile boolean shouldStop = false;
public static void main(String[] args) throws InterruptedException {
Thread t = new Thread(() -> {
while (!shouldStop) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
});
t.start();
// 模拟父线程执行一段时间后需要中断子线程
Thread.sleep(5000);
shouldStop = true;
}
}
```
在上面的代码中,我们创建了一个子线程t,它会每隔1秒钟睡眠一次,然后检查shouldStop变量来判断是否应该停止执行。在父线程中,我们等待5秒钟后将shouldStop设置为true,以便中断子线程的执行。
在子线程中,我们使用了try-catch块来捕获InterruptedException异常,并在catch块中重置中断标志。这是因为当一个线程调用sleep()等方法时,如果被中断了,会抛出InterruptedException异常,此时需要重置中断标志以便后续处理。在实际应用中,可以根据具体情况来决定是否需要捕获InterruptedException异常。
用Java代码编写下面程序:分别采用临界区与Lock加锁方式完成两个子线程之间的互斥。在主线程中使用thread创建两个子线程,通过synchronized构造临界区并使两个子线程互斥使用打印进程。采用Lock加锁方式使两个子线程互斥使用全局变量count。
在Java中,我们可以使用synchronized关键字来实现临界区(Lock-free coding)的互斥访问,以及`java.util.concurrent.locks`包下的Lock接口来实现更精细的控制。下面是使用这两种方式实现的示例代码:
首先,使用synchronized关键字(临界区):
```java
public class SynchronizedExample {
private int count = 0;
public void printProcess(int id) {
synchronized (this) {
System.out.println("Thread " + id + " started - " + count);
// 省略实际操作,这里仅模拟时间消耗
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Thread " + id + " finished - " + count);
}
}
public static void main(String[] args) {
SynchronizedExample example = new SynchronizedExample();
Thread t1 = new Thread(() -> example.printProcess(1));
Thread t2 = new Thread(() -> example.printProcess(2));
t1.start();
t2.start();
}
}
```
接下来,使用ReentrantLock实现Lock方式:
```java
import java.util.concurrent.locks.ReentrantLock;
public class LockExample {
private int count = 0;
private ReentrantLock lock = new ReentrantLock();
public void printProcess(int id) {
lock.lock();
try {
System.out.println("Thread " + id + " started - " + count);
// 模拟时间消耗
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Thread " + id + " finished - " + count);
} finally {
lock.unlock();
}
}
public static void main(String[] args) {
LockExample example = new LockExample();
Thread t1 = new Thread(() -> example.printProcess(1));
Thread t2 = new Thread(() -> example.printProcess(2));
t1.start();
t2.start();
}
}
```
阅读全文
相关推荐
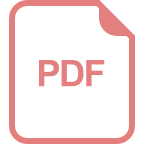
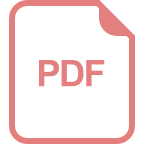
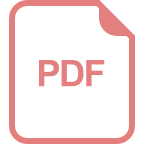
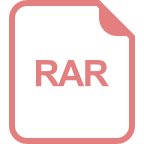
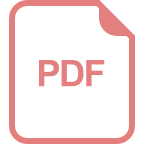
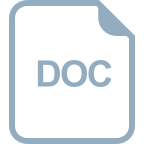









