node.js koa 设置response header
时间: 2024-04-06 22:09:19 浏览: 25
在koa中设置response header可以使用ctx.set()方法,例如:
```javascript
const Koa = require('koa');
const app = new Koa();
app.use(async (ctx) => {
ctx.set('Content-Type', 'text/plain');
ctx.body = 'Hello World';
});
app.listen(3000);
```
在上面的例子中,我们在response header中设置了Content-Type为text/plain。可以通过调用ctx.set()方法来设置其他的response header,例如:
```javascript
ctx.set('Content-Disposition', 'attachment; filename="file.txt"');
```
这将在response header中设置Content-Disposition为attachment,并指定文件名为file.txt。
相关问题
node.js koa-sendfile的使用方法
使用koa-sendfile可以很方便地将文件发送给客户端。以下是使用koa-sendfile的步骤:
1. 安装koa-sendfile
```
npm install koa-sendfile
```
2. 引入koa-sendfile
```
const sendfile = require('koa-sendfile');
```
3. 使用sendfile函数发送文件
```
router.get('/download', async (ctx, next) => {
const path = '/path/to/file';
await sendfile(ctx, path);
});
```
其中,第一个参数是koa的上下文对象,第二个参数是要发送的文件的路径。
4. 可选项
koa-sendfile还有一些可选项,可以根据需要设置:
- root:文件根目录,默认为当前工作目录。
- maxage:缓存时间,单位为毫秒,默认为0,表示不缓存。
- hidden:是否发送隐藏文件,默认为false。
- index:默认文件名,默认为'index.html'。
- gzip:是否开启gzip压缩,默认为true。
- brotli:是否开启brotli压缩,默认为false。
```
await sendfile(ctx, path, {
root: '/path/to/root',
maxage: 1000 * 60 * 60 * 24, // 1 day
hidden: true,
index: 'default.html',
gzip: false,
brotli: true
});
```
node.js koa2-cors 设置 Access-Control-Allow-Origin
要设置 Access-Control-Allow-Origin,可以使用 koa2-cors 中间件。Koa2-cors 是一个 Koa2 中间件,用于处理跨域请求。
以下是如何使用 koa2-cors 中间件来设置 Access-Control-Allow-Origin:
1. 安装 koa2-cors:
```
npm install koa2-cors --save
```
2. 在 Koa2 应用程序中使用 koa2-cors 中间件:
```javascript
const Koa = require('koa');
const cors = require('koa2-cors');
const app = new Koa();
// 使用 koa2-cors 中间件
app.use(cors({
origin: '*', // 允许任意源
credentials: true // 允许带上 cookie
}));
// 其他中间件和路由
app.use(/* ... */);
app.use(/* ... */);
app.use(/* ... */);
app.listen(3000);
```
在上面的代码中,我们传递了一个配置对象给 koa2-cors 中间件。我们设置 origin 为 *,表示允许任意源发起跨域请求。我们也设置了 credentials 为 true,表示允许带上 cookie。
通过这样设置,就可以在 Koa2 应用程序中设置 Access-Control-Allow-Origin。
相关推荐
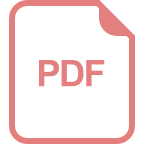
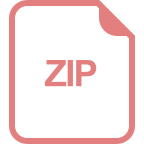














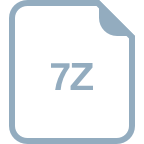