单片机unicode编码转中文C语言程序
时间: 2023-09-19 14:07:03 浏览: 184
在单片机中,如果需要将Unicode编码转换成中文,可以使用一些开源的库函数来实现。下面是一种可以在多种单片机平台上运行的实现方法。
首先,需要在C语言中定义一个包含所有中文字符的数组,例如GB2312编码的中文字库,可以使用如下代码:
```c
const unsigned char gb_font[] = {
// 从0xA1开始的中文字符,共有94个字符
// 每个字符占用16个字节,每个字节高位为0,低位为实际的字节值
// 根据GB2312编码,第一个字节的范围是0xA1-0xF7,第二个字节的范围是0xA1-0xFE
// 因此,字库的大小为(0xF7-0xA1+1) * (0xFE-0xA1+1) * 16 = 67680个字节
// 这里只列出前面几个字的数据,具体数据可以参考字库文件
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // 空格
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // !
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // "
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // #
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 // $
// 其他字符的数据省略
};
```
接下来,可以使用Unicode编码的字符数组来表示中文字符串,然后使用字库中对应的汉字来进行转换。具体实现可以参考以下代码:
```c
// 中文字符串
const unsigned short chinese_str[] = {0x4E2D, 0x6587, 0x5B57, 0x7B26};
// 将Unicode编码的中文字符串转换成GB2312编码的中文字符串
void unicode_to_chinese(unsigned short *unicode_str, char *chinese_str)
{
int i;
for (i = 0; unicode_str[i] != 0; i++) {
unsigned short ch = unicode_str[i];
// 在字库中查找对应的汉字
// 假设字库中每个汉字占用16个字节,字库地址为0x80000000
unsigned char *font = gb_font + (ch - 0xA1A1) * 16;
// 将汉字转换成GB2312编码
chinese_str[i * 2] = font[0];
chinese_str[i * 2 + 1] = font[1];
}
chinese_str[i * 2] = '\0';
}
int main(void)
{
char chinese_str[sizeof(chinese_str) * 2 + 1];
unicode_to_chinese(chinese_str, chinese_str);
printf("中文字符串:%s\n", chinese_str);
return 0;
}
```
需要注意的是,上述代码中的字库地址和字库格式等都需要根据具体情况进行调整。此外,如果使用其他单片机平台,可能需要使用其他库函数或者自己实现字库解析和编码转换等功能。
阅读全文
相关推荐
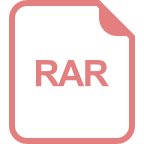
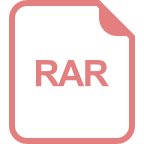
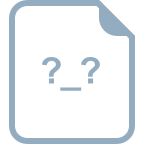
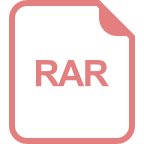
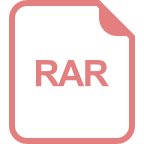
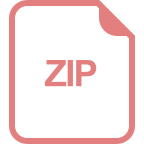
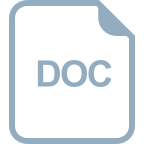
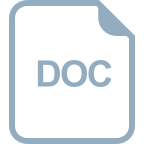

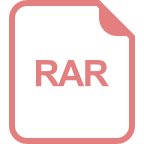
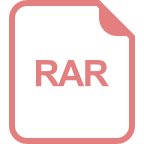
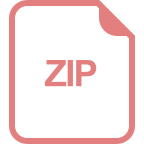
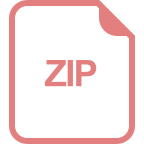
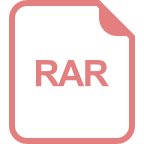
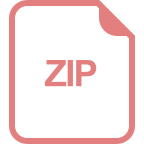
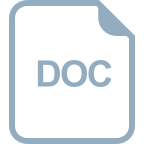
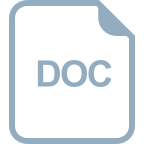