next_permutation怎么用
时间: 2023-05-25 16:06:27 浏览: 65
next_permutation 是一个 C++ 中的 STL 函数,用于获取下一个排列顺序。它的使用方式如下:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
int arr[] = {1, 2, 3};
int n = sizeof(arr)/sizeof(arr[0]);
do {
for (int i=0; i<n; i++)
cout << arr[i] << " ";
cout << endl;
} while (next_permutation(arr, arr+n));
return 0;
}
```
解释:
- 首先要 include <algorithm> 头文件,该头文件中包括了 next_permutation 函数。
- 在程序中定义了一个数组 arr,它的初始排列顺序为 {1, 2, 3}。同时,变量 n 表示数组的长度。
- 应用了 do…while 循环,用于不断获取下一个排列顺序,并输出到屏幕上。当获取到最后一个排列顺序时,next_permutation 函数返回 false,循环结束。
- 在 do…while 循环中,使用 next_permutation 函数对 arr 数组进行排列。排列结果被输出到屏幕上。
相关问题
next_permutation
next_permutation is a function in the C++ STL (Standard Template Library) that generates the next lexicographically greater permutation of a sequence. It modifies the sequence in place, and if there is no greater permutation, it returns false.
Here is an example usage of next_permutation:
```
#include <algorithm>
#include <iostream>
#include <vector>
int main() {
std::vector<int> v = {1, 2, 3};
do {
for (int i : v) {
std::cout << i << ' ';
}
std::cout << '\n';
} while (std::next_permutation(v.begin(), v.end()));
return 0;
}
```
This program generates all permutations of the sequence {1, 2, 3}:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
```
The function std::next_permutation is called in the while loop condition to generate the next permutation. The loop continues until there is no greater permutation left.
next_permutation 怎么用
next_permutation 是一个STL函数,用于对一个序列进行全排列。它可以用于排列算法中,对于排列的生成,可以使用它来生成下一个排列。
使用方法:
1. 首先需要包含头文件<algorithm>。
2. next_permutation 函数的参数是一个区间,因此需要传递两个迭代器,表示区间的开始和结束。
3. 该函数会对区间进行全排列,并返回 true,如果区间已经是最后一个排列,则返回 false。
示例代码:
```cpp
#include <iostream>
#include <algorithm>
using namespace std;
int main() {
int a[] = {1, 2, 3};
do {
cout << a[0] << " " << a[1] << " " << a[2] << endl;
} while (next_permutation(a, a + 3));
return 0;
}
```
输出结果:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
```
该程序对数组 {1, 2, 3} 进行全排列,并输出结果。
阅读全文
相关推荐
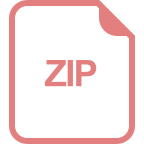
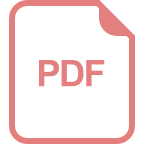




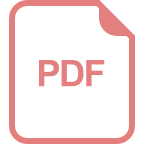
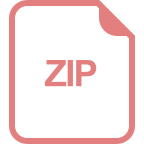
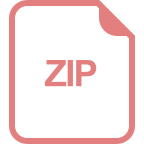