题目描述 在下面的程序中,在begin和end之间定义一个计算最高分的方法。 import java.util.Scanner; /** * * 实现计算5名学生成绩的最高分 * */ class StudentsBiz { /** * 求最高分,方法名calMax() * @param scores 参赛成绩数组 */ /************ begin ************/ /************* end ************? } public class Main { /** * 调用带数组参数的方法 */ public static void main(String[] args) { StudentsBiz st = new StudentsBiz(); int[] scores=new int[5]; Scanner input = new Scanner(System.in); //System.out.println("请输入五名参赛者的成绩:"); for(int i=0;i<5;i++){ scores[i] = input.nextInt(); } int maxScore=st.calMax(scores); System.out.println("最高分:"+ maxScore); } } 输入 输入5个学生的成绩。成绩为int类型数据。 输出 输出最高分。 样例输入 Copy 50 60 70 80 90 样例输出 Copy 最高分:90
时间: 2023-07-01 19:17:56 浏览: 107
在begin和end之间定义一个计算最高分的方法,可以如下实现:
```
import java.util.Scanner;
/**
* 实现计算5名学生成绩的最高分
*/
class StudentsBiz {
/**
* 求最高分,方法名calMax()
*
* @param scores 参赛成绩数组
*/
int calMax(int[] scores) {
int maxScore = scores[0]; // 假设第一个学生成绩最高
for (int i = 1; i < scores.length; i++) {
if (scores[i] > maxScore) {
maxScore = scores[i]; // 更新最高分
}
}
return maxScore; // 返回最高分
}
}
public class Main {
/**
* 调用带数组参数的方法
*/
public static void main(String[] args) {
StudentsBiz st = new StudentsBiz();
int[] scores = new int[5];
Scanner input = new Scanner(System.in);
// System.out.println("请输入五名参赛者的成绩:");
for (int i = 0; i < 5; i++) {
scores[i] = input.nextInt();
}
int maxScore = st.calMax(scores);
System.out.println("最高分:" + maxScore);
input.close();
}
}
```
该程序使用calMax方法计算最高分,参数为参赛成绩数组,返回值为最高分。在main方法中,先读取5个学生成绩存入数组中,然后调用calMax方法计算最高分并输出。需要注意的是,使用Scanner读取输入后,需要关闭输入流,可以使用`input.close()`方法关闭。
阅读全文
相关推荐
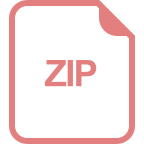
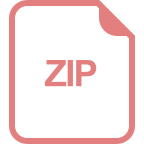
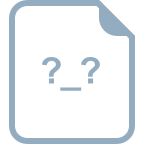
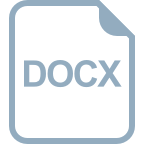
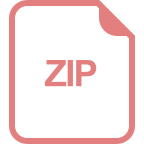
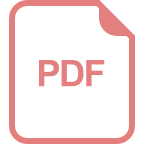
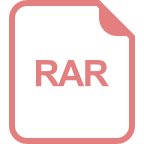
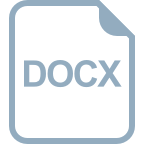
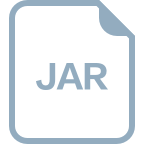
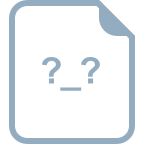
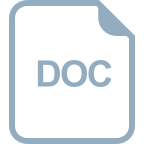
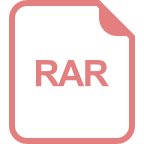
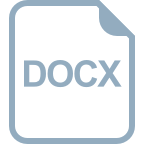
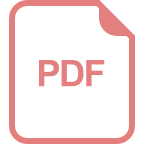
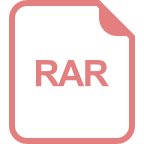
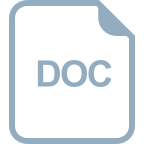
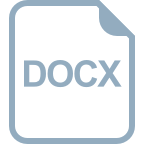