import java.util.List; import java.util.stream.Collectors; @Service public class EmpService { @Resource private EmpMapper empMapper; @Resource private DeptMapper deptMapper; public List<Emp> listEmp(Emp emp) { List<Emp> empList = empMapper.selectList(Wrappers.<Emp>lambdaQuery() .orderByAsc(Emp::getId) ); if (!empList.isEmpty()) { List<Long> deptIdList = empList.stream().map(Emp::getDeptId).collect(Collectors.toList()); List<Dept> deptList = deptMapper.selectBatchIds(deptIdList); for (Emp empItem : empList) { empItem.setDept(deptList.stream().filter(dept -> dept.getId().equals(empItem.getDeptId())).findAny().orElse(null)); } } return empList; } public Emp getEmpById(String id) { return empMapper.selectById(id); } public int insertEmp(Emp emp) { return empMapper.insert(emp); } public int updateEmp(Emp emp) { return empMapper.updateById(emp); } public int deleteEmp(String id) { return empMapper.deleteById(id); } }
时间: 2024-04-10 14:32:32 浏览: 23
这段代码定义了一个名为"EmpService"的服务类,用于处理员工信息的业务逻辑。
注解包括:
- "@Service":标识该类是一个服务类,用于将其纳入Spring容器进行管理。
属性包括:
- "empMapper":使用了"@Resource"注解,表示该属性会从Spring容器中自动注入一个"EmpMapper"的实例。
- "deptMapper":使用了"@Resource"注解,表示该属性会从Spring容器中自动注入一个"DeptMapper"的实例。
方法包括:
- "listEmp":查询员工信息列表。首先使用"empMapper.selectList()"方法查询所有员工信息,并按照id升序排序。然后通过stream操作将得到的员工列表中的deptId提取出来,再通过deptMapper.selectBatchIds()方法批量查询对应的部门信息。最后,通过循环遍历员工列表,将对应的部门信息设置到每个员工对象中,并返回员工列表。
- "getEmpById":根据员工id查询员工信息。使用"empMapper.selectById()"方法根据id查询员工信息,并返回结果。
- "insertEmp":插入员工信息。使用"empMapper.insert()"方法将员工信息插入到数据库中,并返回插入操作影响的行数。
- "updateEmp":更新员工信息。使用"empMapper.updateById()"方法根据员工id更新员工信息,并返回更新操作影响的行数。
- "deleteEmp":删除员工信息。使用"empMapper.deleteById()"方法根据员工id删除员工信息,并返回删除操作影响的行数。
该服务类提供了对员工信息的增删改查等常用操作方法,通过使用"EmpMapper"和"DeptMapper"来与数据库进行交互。
相关推荐
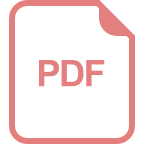
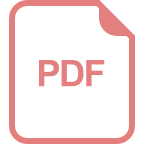















