在androidstudio中创建一个显示天气日期温度的页面布局
时间: 2023-10-05 22:05:23 浏览: 104
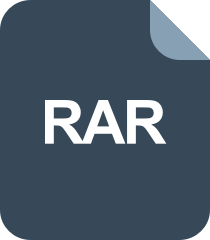
android studio开发的天气预报
首先,你需要在布局文件中添加一个TextView来显示日期、温度等信息。然后,你可以使用一些现成的库来获取天气数据,例如OpenWeatherMap API。
以下是一个简单的示例布局文件:
```
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/dateTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp"
android:textColor="@color/black"
android:text="Today's Date"
android:layout_marginTop="16dp"
android:layout_centerHorizontal="true"/>
<TextView
android:id="@+id/temperatureTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="48sp"
android:textColor="@color/red"
android:text="Temperature"
android:layout_below="@id/dateTextView"
android:layout_centerHorizontal="true"
android:layout_marginTop="32dp"/>
</RelativeLayout>
```
在这个布局文件中,我们添加了两个TextView。第一个TextView用于显示日期,第二个TextView用于显示温度。我们还使用了RelativeLayout来控制它们的位置。
接下来,你需要在你的Activity中使用这个布局文件,并设置日期和温度值。你可以使用Java中的SimpleDateFormat来格式化日期,然后使用OpenWeatherMap API来获取天气数据。以下是一个简单的示例代码:
```
public class WeatherActivity extends AppCompatActivity {
private TextView dateTextView, temperatureTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_weather);
dateTextView = findViewById(R.id.dateTextView);
temperatureTextView = findViewById(R.id.temperatureTextView);
// 获取当前日期并格式化
SimpleDateFormat dateFormat = new SimpleDateFormat("EEE, MMM d");
String currentDate = dateFormat.format(new Date());
// 获取天气数据并设置温度值
WeatherApiClient weatherApiClient = new WeatherApiClient();
weatherApiClient.getTemperature(new WeatherApiClient.Callback() {
@Override
public void onSuccess(double temperature) {
temperatureTextView.setText(String.format("%.1f℃", temperature));
}
@Override
public void onError(String message) {
temperatureTextView.setText("N/A");
}
});
// 设置日期值
dateTextView.setText(currentDate);
}
}
```
在这个示例代码中,我们首先获取当前日期并使用SimpleDateFormat格式化。然后,我们使用WeatherApiClient类来获取天气数据,并在回调中设置温度值。最后,我们设置日期值。请注意,这里使用了一个回调来处理异步获取天气数据的过程。
这只是一个简单的示例,你需要根据你的具体需求来修改和完善它。
阅读全文
相关推荐
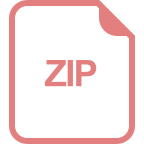
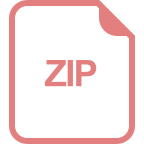
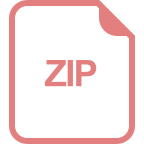
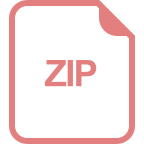
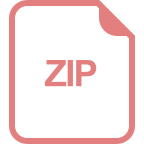
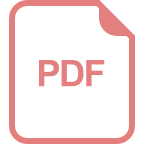
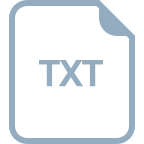
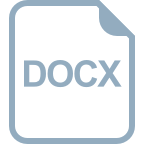
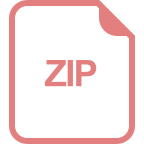
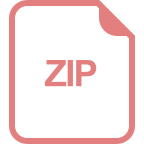
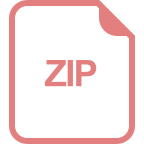
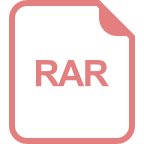
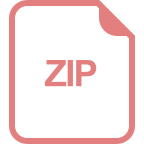
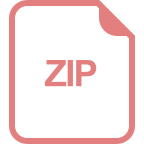
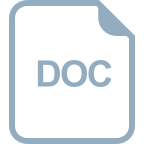
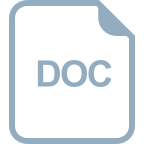
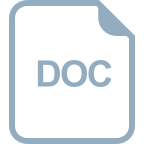