对家谱管理进行简单的模拟,以实现查看祖先和子孙个人信息,插入家族 成员,删除家族成员的功能。 项目功能要求: 本项目的实质是完成家谱成员信息的建立,查找,插入,修改,删除等功能, 可以首先定义家族成员数据结构,然后将每个功能作为一个成员函数来完成 对数据的操作,最后完成主函数以验证各个函数功能并得到运行结果。请给出完整的C语言代码
时间: 2024-04-07 13:30:29 浏览: 137
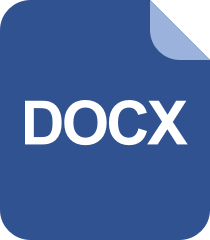
家谱课程设计.docx
以下是一个简单的C语言代码示例,实现了家谱管理的基本功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LENGTH 50
typedef struct {
char name[MAX_NAME_LENGTH];
struct Node* children;
} Node;
Node* createNode(char* name) {
Node* newNode = (Node*) malloc(sizeof(Node));
strcpy(newNode->name, name);
newNode->children = NULL;
return newNode;
}
void insertChild(Node* parent, char* name) {
Node* child = createNode(name);
child->children = parent->children;
parent->children = child;
}
void deleteChild(Node* parent, char* name) {
Node* current = parent->children;
Node* previous = NULL;
while (current != NULL) {
if (strcmp(current->name, name) == 0) {
if (previous == NULL) {
parent->children = current->children;
} else {
previous->children = current->children;
}
free(current);
return;
}
previous = current;
current = current->children;
}
}
void printDescendants(Node* node, int level) {
int i;
for (i = 0; i < level; i++) {
printf(" ");
}
printf("%s\n", node->name);
Node* current = node->children;
while (current != NULL) {
printDescendants(current, level + 1);
current = current->children;
}
}
int main() {
Node* root = createNode("家谱");
insertChild(root, "祖先1");
insertChild(root, "祖先2");
insertChild(root->children, "子孙1");
insertChild(root->children, "子孙2");
printf("家谱成员信息:\n");
printDescendants(root, 0);
deleteChild(root->children, "子孙1");
printf("\n删除后的家谱成员信息:\n");
printDescendants(root, 0);
return 0;
}
```
这段代码中,我们使用了一个`Node`结构体来表示家族成员,每个节点包含一个姓名和一个指向子节点的指针。通过使用`createNode`函数创建新的节点,`insertChild`函数插入子节点,`deleteChild`函数删除子节点,并使用`printDescendants`函数打印祖先和子孙个人信息。
在主函数中,我们创建了一个名为"家谱"的根节点,并插入了一些祖先和子孙的信息。然后,我们打印出家谱成员的信息,并删除了一个子孙节点后再次打印家谱成员的信息。
请注意,此代码示例只是一个简单的模拟,并没有涉及到文件存储和用户输入。在实际应用中,你可能需要扩展代码来支持更多功能,并根据具体需求进行适当的修改。
阅读全文
相关推荐
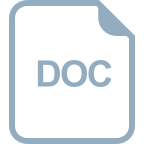
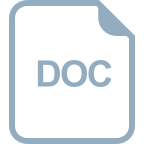
















