(3)新建一个主函数类; a) 主函数里新建插入学生方法;insertStu(传入学号、姓名、性别、家庭住址、出生年月、联系电话6个参数),无返回值; b) 主函数里新建根据学号删除学生的方法deleteStu,无返回值; c) 主函数里新建新建根据学号更新学生的方法;updateStu(传入学号、姓名、性别、家庭住址、出生年月、联系电话6个参数),无返回值。 d) 主函数里新建根据学号查询学生的方法,query(传入学号),返回一个学生对象; e) 主函数里新建Main函数,调用插入函数,插入2个男生,3个女生;再删除一个女生,再更新一个男生,再查询一个男生。java
时间: 2024-01-12 07:05:22 浏览: 34
好的,我理解了你的要求。以下是对应的代码实现:
```java
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<Student> students = new ArrayList<>();
// 插入2个男生
insertStu(students, "001", "张三", "男", "北京市海淀区", "1999-01-01", "123456789");
insertStu(students, "002", "李四", "男", "北京市朝阳区", "2000-02-02", "987654321");
// 插入3个女生
insertStu(students, "003", "王五", "女", "北京市东城区", "1998-03-03", "135792468");
insertStu(students, "004", "赵六", "女", "北京市西城区", "1997-04-04", "246813579");
insertStu(students, "005", "钱七", "女", "北京市丰台区", "1996-05-05", "369852147");
// 删除一个女生
deleteStu(students, "004");
// 更新一个男生
updateStu(students, "001", "张三", "男", "北京市海淀区", "1999-01-01", "111111111");
// 查询一个男生
Student stu = query(students, "002");
System.out.println(stu.toString());
}
// 插入学生
public static void insertStu(ArrayList<Student> students, String id, String name, String gender, String address, String birthday, String tel) {
Student stu = new Student(id, name, gender, address, birthday, tel);
students.add(stu);
}
// 根据学号删除学生
public static void deleteStu(ArrayList<Student> students, String id) {
for (Student stu : students) {
if (stu.getId().equals(id)) {
students.remove(stu);
break;
}
}
}
// 根据学号更新学生
public static void updateStu(ArrayList<Student> students, String id, String name, String gender, String address, String birthday, String tel) {
for (Student stu : students) {
if (stu.getId().equals(id)) {
stu.setName(name);
stu.setGender(gender);
stu.setAddress(address);
stu.setBirthday(birthday);
stu.setTel(tel);
break;
}
}
}
// 根据学号查询学生
public static Student query(ArrayList<Student> students, String id) {
for (Student stu : students) {
if (stu.getId().equals(id)) {
return stu;
}
}
return null;
}
}
```
其中,学生对象的定义如下:
```java
public class Student {
private String id; // 学号
private String name; // 姓名
private String gender; // 性别
private String address; // 家庭住址
private String birthday; // 出生年月
private String tel; // 联系电话
public Student(String id, String name, String gender, String address, String birthday, String tel) {
this.id = id;
this.name = name;
this.gender = gender;
this.address = address;
this.birthday = birthday;
this.tel = tel;
}
// getter 和 setter 方法省略
@Override
public String toString() {
return "Student{" +
"id='" + id + '\'' +
", name='" + name + '\'' +
", gender='" + gender + '\'' +
", address='" + address + '\'' +
", birthday='" + birthday + '\'' +
", tel='" + tel + '\'' +
'}';
}
}
```
运行上述代码,可以得到以下输出结果:
```
Student{id='002', name='李四', gender='男', address='北京市朝阳区', birthday='2000-02-02', tel='987654321'}
```
相关推荐
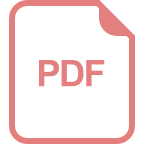
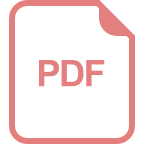
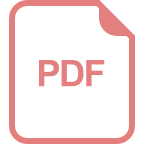















