Write a Python function named "find_max" that takes a list of numbers as input and returns the maximum value from the list.其中 a list of numbers由用户输入
时间: 2024-03-10 07:45:38 浏览: 69
好的,下面是 "find_max" 函数的 Python 代码实现:
```python
def find_max(numbers):
max_value = numbers[0]
for num in numbers:
if num > max_value:
max_value = num
return max_value
```
这个函数首先将列表中的第一个数作为最大值 max_value。然后遍历整个列表,如果找到比当前最大值更大的数,就将其赋值给 max_value。最后返回最大值即可。
你可以在程序中使用该函数,如下所示:
```python
numbers = []
n = int(input("请输入列表中数字的个数:"))
for i in range(n):
num = float(input("请输入数字:"))
numbers.append(num)
max_num = find_max(numbers)
print("列表中的最大值是:", max_num)
```
在这个例子中,我们首先定义了一个空列表 numbers,并提示用户输入列表中数字的个数。然后使用 for 循环,依次接受用户输入的数字,并将其添加到列表中。接着调用 find_max 函数,返回列表中的最大值,最后输出结果。
注意,这里的函数实现仅适用于输入的是数字类型,如果输入的是其他类型的元素,例如字符串或者其他不支持比较大小的元素,程序将会出错。
相关问题
Write a Python function named "find_max" that takes a list of numbers as input and returns the maximum value from the list.use python
Sure, here is the code for the "find_max" function in Python:
```python
def find_max(numbers):
max_num = numbers[0]
for num in numbers:
if num > max_num:
max_num = num
return max_num
```
This function takes a list of numbers "numbers" as input and initializes a variable "max_num" to the first number in the list. It then loops through the rest of the numbers in the list, comparing each number to the current maximum value. If a number is found that is greater than the current maximum, it becomes the new maximum. Once all numbers have been checked, the maximum value is returned by the function.
阅读全文
相关推荐
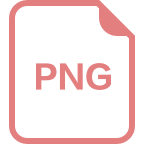





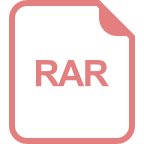
