linux tcpdump C++ 代码
时间: 2023-10-21 07:04:54 浏览: 149
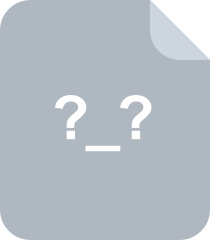
tcpdump 源代码

以下是一个简单的使用tcpdump的C代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#include <netinet/ip.h>
#include <netinet/tcp.h>
#include <pcap.h>
void print_packet(const u_char *packet, struct pcap_pkthdr packet_header) {
struct iphdr *ip_header = (struct iphdr*)(packet + sizeof(struct ethhdr));
unsigned short ip_header_length = ip_header->ihl * 4;
struct tcphdr *tcp_header = (struct tcphdr*)(packet + sizeof(struct ethhdr) + ip_header_length);
printf("Source IP: %s\n", inet_ntoa(*(struct in_addr*)&ip_header->saddr));
printf("Destination IP: %s\n", inet_ntoa(*(struct in_addr*)&ip_header->daddr));
printf("Source Port: %d\n", ntohs(tcp_header->source));
printf("Destination Port: %d\n", ntohs(tcp_header->dest));
printf("Sequence Number: %u\n", ntohl(tcp_header->seq));
printf("Acknowledgement Number: %u\n", ntohl(tcp_header->ack_seq));
printf("Flags: ");
if (tcp_header->urg) printf("U");
if (tcp_header->ack) printf("A");
if (tcp_header->psh) printf("P");
if (tcp_header->rst) printf("R");
if (tcp_header->syn) printf("S");
if (tcp_header->fin) printf("F");
printf("\n");
printf("Data Length: %d\n", packet_header.len - sizeof(struct ethhdr) - ip_header_length - tcp_header->doff * 4);
}
int main(int argc, char **argv) {
char *device = "eth0";
char error_buffer[PCAP_ERRBUF_SIZE];
pcap_t *handle = pcap_open_live(device, BUFSIZ, 1, 1000, error_buffer);
if (handle == NULL) {
fprintf(stderr, "Couldn't open device %s: %s\n", device, error_buffer);
exit(EXIT_FAILURE);
}
struct bpf_program filter;
char *filter_exp = "tcp and port 80";
if (pcap_compile(handle, &filter, filter_exp, 0, PCAP_NETMASK_UNKNOWN) == -1) {
fprintf(stderr, "Couldn't parse filter %s: %s\n", filter_exp, pcap_geterr(handle));
exit(EXIT_FAILURE);
}
if (pcap_setfilter(handle, &filter) == -1) {
fprintf(stderr, "Couldn't install filter %s: %s\n", filter_exp, pcap_geterr(handle));
exit(EXIT_FAILURE);
}
struct pcap_pkthdr packet_header;
const u_char *packet;
while ((packet = pcap_next(handle, &packet_header)) != NULL) {
print_packet(packet, packet_header);
}
pcap_close(handle);
return 0;
}
```
该代码使用pcap库捕获网络数据包并解析TCP数据包的IP地址、端口号、序列号、确认号、标记和数据长度。它还使用BPF过滤器仅捕获TCP端口80的数据包。
阅读全文
相关推荐




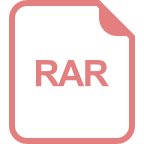
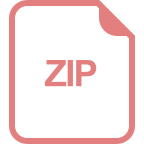
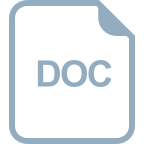
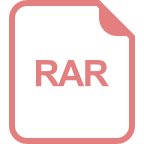
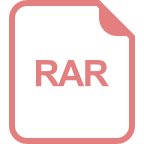
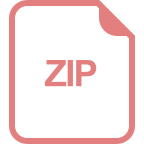
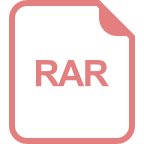
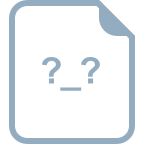
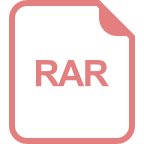
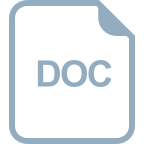
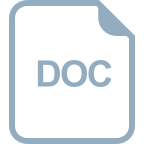
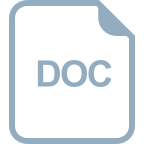
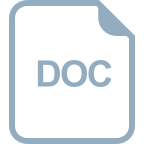
