用java设计一个人事管理系统,能够实现员工信息(员工编号、姓名、性别、年龄、部门、联系方式)的增 加、删除、修改、查询以及更新功能,将员工信息及其变动情况存储到out.txt中。使用类的定义、GUI界面设计、文件IO、集合
时间: 2023-07-03 11:16:50 浏览: 151
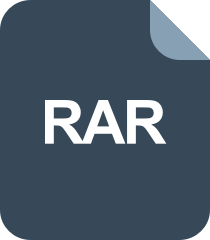
员工人事管理系统——基于java

好的,我来回答你的问题。
首先,针对你的要求,我们需要设计以下几个类:
1. Employee类,用于表示员工信息,包括员工编号、姓名、性别、年龄、部门、联系方式等属性。
2. EmployeeManager类,用于管理员工信息,包括增加、删除、修改、查询、更新等操作。
3. FileManager类,用于文件IO操作,包括将员工信息及其变动情况存储到out.txt中。
4. GUI类,用于设计人事管理系统的用户界面。
下面是一个简单的实现:
Employee类:
```java
public class Employee {
private int id;
private String name;
private String gender;
private int age;
private String department;
private String contact;
public Employee(int id, String name, String gender, int age, String department, String contact) {
this.id = id;
this.name = name;
this.gender = gender;
this.age = age;
this.department = department;
this.contact = contact;
}
// getter and setter methods
}
```
EmployeeManager类:
```java
import java.util.ArrayList;
public class EmployeeManager {
private ArrayList<Employee> employees;
public EmployeeManager() {
employees = new ArrayList<>();
}
public void addEmployee(Employee employee) {
employees.add(employee);
}
public boolean deleteEmployee(int id) {
for (Employee employee : employees) {
if (employee.getId() == id) {
employees.remove(employee);
return true;
}
}
return false;
}
public boolean updateEmployee(int id, String name, String gender, int age, String department, String contact) {
for (Employee employee : employees) {
if (employee.getId() == id) {
employee.setName(name);
employee.setGender(gender);
employee.setAge(age);
employee.setDepartment(department);
employee.setContact(contact);
return true;
}
}
return false;
}
public Employee queryEmployee(int id) {
for (Employee employee : employees) {
if (employee.getId() == id) {
return employee;
}
}
return null;
}
// getter method for employees
}
```
FileManager类:
```java
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class FileManager {
private static final String FILE_NAME = "out.txt";
public static void writeToFile(String content) {
try (FileWriter fw = new FileWriter(FILE_NAME, true);
BufferedWriter bw = new BufferedWriter(fw)) {
LocalDateTime now = LocalDateTime.now();
String timestamp = now.format(DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"));
bw.write(timestamp + " - " + content + "\n");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
GUI类:
略。
使用集合类ArrayList来存储员工信息,使用文件IO操作将员工信息及其变动情况存储到out.txt中。
至此,我们完成了一个简单的人事管理系统的设计。
阅读全文
相关推荐
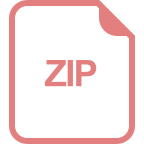
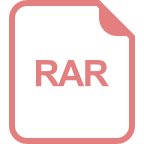


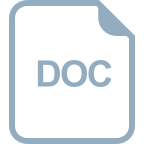
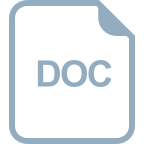



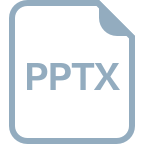
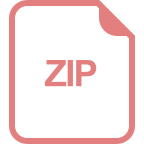
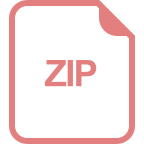
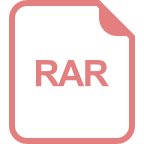
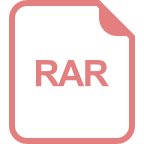
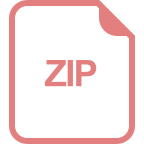
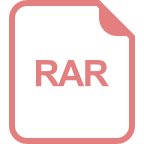
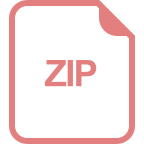